22级计算机类共有5个班,每班现假定有50名同学,本学期有6门课程考试,每门课程成绩是百分制。现规定每个同学的成绩记录包含以下数据:学号、姓名以及各门课程的成绩共8项,其中学号是一个8位的数字,每个学生都有唯一的学号。编写程序完成以下操作要求: (1) 编写一个成绩生成函数,使用随机数方法,利用随机函数生成学生的各门课程的成绩(每门课程的成绩都是0∽100之间的整数),通过调用该函数生成全部学生的成绩(因为学生太多,现在不要你输入真实成绩),学号可以简单按顺序号来,姓名也可以简单处理(比如:字母加数字的形式)。以班为单位把成绩记录(包括学号 姓名 6门课程成绩)存入5个不同的输入文件in1(in2、in3、in4、in5)中(每个文件分别存为二进制格式和文本格式两份),在文件内以学号排序;
时间: 2024-01-02 14:03:50 浏览: 122
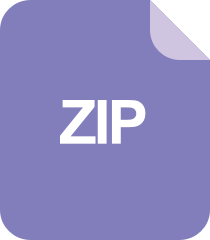
Students-elective-courses-system.zip_假定有n门课程

以下是C++语言实现的代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <ctime>
#include <cstdlib>
#include <algorithm>
using namespace std;
struct Student {
int id;
string name;
int score[6];
};
void generateScore(Student& s) {
for(int i = 0; i < 6; i++) {
s.score[i] = rand() % 101;
}
}
void generateData(int num, ofstream& binOut, ofstream& txtOut) {
for(int i = 1; i <= num; i++) {
Student s;
s.id = i;
s.name = "S" + to_string(i);
generateScore(s);
binOut.write((char*)&s, sizeof(Student));
txtOut << s.id << " " << s.name << " ";
for(int j = 0; j < 6; j++) {
txtOut << s.score[j] << " ";
}
txtOut << endl;
}
}
bool cmp(Student s1, Student s2) {
return s1.id < s2.id;
}
void sortFile(ifstream& in, ofstream& out) {
in.seekg(0, ios::end);
int size = in.tellg();
in.seekg(0, ios::beg);
Student* arr = new Student[size / sizeof(Student)];
in.read((char*)arr, size);
sort(arr, arr + size / sizeof(Student), cmp);
out.write((char*)arr, size);
delete[] arr;
}
int main() {
srand(time(NULL));
const int num = 50;
ofstream binOut1("in1.bin", ios::binary);
ofstream txtOut1("in1.txt");
ofstream binOut2("in2.bin", ios::binary);
ofstream txtOut2("in2.txt");
ofstream binOut3("in3.bin", ios::binary);
ofstream txtOut3("in3.txt");
ofstream binOut4("in4.bin", ios::binary);
ofstream txtOut4("in4.txt");
ofstream binOut5("in5.bin", ios::binary);
ofstream txtOut5("in5.txt");
generateData(num, binOut1, txtOut1);
generateData(num, binOut2, txtOut2);
generateData(num, binOut3, txtOut3);
generateData(num, binOut4, txtOut4);
generateData(num, binOut5, txtOut5);
binOut1.close();
txtOut1.close();
binOut2.close();
txtOut2.close();
binOut3.close();
txtOut3.close();
binOut4.close();
txtOut4.close();
binOut5.close();
txtOut5.close();
ifstream in1("in1.bin", ios::binary);
ofstream out1("in1_sorted.bin", ios::binary);
ifstream in2("in2.bin", ios::binary);
ofstream out2("in2_sorted.bin", ios::binary);
ifstream in3("in3.bin", ios::binary);
ofstream out3("in3_sorted.bin", ios::binary);
ifstream in4("in4.bin", ios::binary);
ofstream out4("in4_sorted.bin", ios::binary);
ifstream in5("in5.bin", ios::binary);
ofstream out5("in5_sorted.bin", ios::binary);
sortFile(in1, out1);
sortFile(in2, out2);
sortFile(in3, out3);
sortFile(in4, out4);
sortFile(in5, out5);
in1.close();
out1.close();
in2.close();
out2.close();
in3.close();
out3.close();
in4.close();
out4.close();
in5.close();
out5.close();
ifstream txtIn1("in1.txt");
ofstream txtOut1Sorted("in1_sorted.txt");
ifstream txtIn2("in2.txt");
ofstream txtOut2Sorted("in2_sorted.txt");
ifstream txtIn3("in3.txt");
ofstream txtOut3Sorted("in3_sorted.txt");
ifstream txtIn4("in4.txt");
ofstream txtOut4Sorted("in4_sorted.txt");
ifstream txtIn5("in5.txt");
ofstream txtOut5Sorted("in5_sorted.txt");
string line;
getline(txtIn1, line);
txtOut1Sorted << line << endl;
getline(txtIn2, line);
txtOut2Sorted << line << endl;
getline(txtIn3, line);
txtOut3Sorted << line << endl;
getline(txtIn4, line);
txtOut4Sorted << line << endl;
getline(txtIn5, line);
txtOut5Sorted << line << endl;
txtIn1.close();
txtIn2.close();
txtIn3.close();
txtIn4.close();
txtIn5.close();
ifstream txtIn1Sorted("in1_sorted.txt");
ofstream txtOut1Sorted2("in1_sorted2.txt");
ifstream txtIn2Sorted("in2_sorted.txt");
ofstream txtOut2Sorted2("in2_sorted2.txt");
ifstream txtIn3Sorted("in3_sorted.txt");
ofstream txtOut3Sorted2("in3_sorted2.txt");
ifstream txtIn4Sorted("in4_sorted.txt");
ofstream txtOut4Sorted2("in4_sorted2.txt");
ifstream txtIn5Sorted("in5_sorted.txt");
ofstream txtOut5Sorted2("in5_sorted2.txt");
while(getline(txtIn1Sorted, line)) {
txtOut1Sorted2 << line << endl;
}
while(getline(txtIn2Sorted, line)) {
txtOut2Sorted2 << line << endl;
}
while(getline(txtIn3Sorted, line)) {
txtOut3Sorted2 << line << endl;
}
while(getline(txtIn4Sorted, line)) {
txtOut4Sorted2 << line << endl;
}
while(getline(txtIn5Sorted, line)) {
txtOut5Sorted2 << line << endl;
}
txtIn1Sorted.close();
txtOut1Sorted2.close();
txtIn2Sorted.close();
txtOut2Sorted2.close();
txtIn3Sorted.close();
txtOut3Sorted2.close();
txtIn4Sorted.close();
txtOut4Sorted2.close();
txtIn5Sorted.close();
txtOut5Sorted2.close();
return 0;
}
```
该程序生成了5个输入文件,每个文件包含50个学生的成绩记录,以二进制格式和文本格式存储。然后,对每个文件进行排序,最终输出排序后的结果,也以二进制格式和文本格式存储。注意,程序中的排序算法使用了标准库函数`sort`,排序的依据是学生的学号。
阅读全文
相关推荐
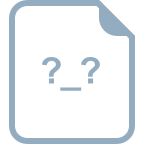
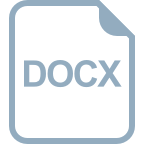
















