c语言实现生产者与消费者问题
时间: 2023-11-06 12:06:02 浏览: 58
生产者与消费者问题可以使用线程和信号量来实现。
以下是一个简单的C语言程序,使用互斥锁和条件变量来解决生产者和消费者问题:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
pthread_mutex_t mutex;
sem_t empty;
sem_t full;
void* producer(void* arg)
{
int item;
while (1) {
item = rand();
sem_wait(&empty);
pthread_mutex_lock(&mutex);
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
printf("Produced item: %d\n", item);
pthread_mutex_unlock(&mutex);
sem_post(&full);
}
}
void* consumer(void* arg)
{
int item;
while (1) {
sem_wait(&full);
pthread_mutex_lock(&mutex);
item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
printf("Consumed item: %d\n", item);
pthread_mutex_unlock(&mutex);
sem_post(&empty);
}
}
int main()
{
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
在该程序中,生产者线程和消费者线程无限循环地执行。生产者线程生成一个随机数并将其放入缓冲区中,然后唤醒消费者线程。消费者线程从缓冲区中获取一个项目并打印出来,然后唤醒生产者线程。
互斥锁用于保护缓冲区的读写操作,而信号量则用于控制缓冲区的空闲和已用空间。当缓冲区已满时,生产者线程被阻塞在 sem_wait(&empty) 处,直到有空间可用。当缓冲区为空时,消费者线程被阻塞在 sem_wait(&full) 处,直到有项目可用。
注意,该程序仅为示例,实际应用中可能需要更复杂的同步机制和错误处理。
相关推荐
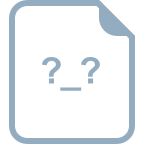
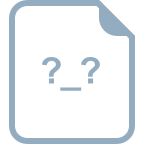
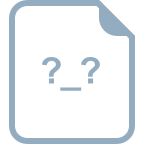













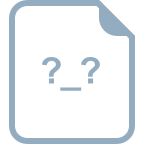
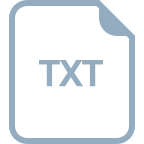