c语言实现生产者多消费者
时间: 2023-08-12 21:08:48 浏览: 119
生产者多消费者问题是一个经典的同步问题,可以使用互斥锁和条件变量来实现。
以下是一个使用C语言实现的生产者多消费者问题:
```c
#include <pthread.h>
#include <stdio.h>
#define BUFFER_SIZE 10
#define PRODUCER_NUM 2
#define CONSUMER_NUM 3
int buffer[BUFFER_SIZE]; // 缓冲区
int in = 0, out = 0; // 缓冲区读写位置
int count = 0; // 缓冲区中的元素数量
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t not_full; // 缓冲区不满的条件变量
pthread_cond_t not_empty; // 缓冲区不空的条件变量
void *producer(void *arg)
{
int id = *(int *)arg;
while (1) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
printf("producer %d: buffer is full, wait...\n", id);
pthread_cond_wait(¬_full, &mutex);
}
buffer[in] = rand();
printf("producer %d: produce item %d\n", id, buffer[in]);
in = (in + 1) % BUFFER_SIZE;
count++;
pthread_cond_signal(¬_empty);
pthread_mutex_unlock(&mutex);
sleep(1);
}
return NULL;
}
void *consumer(void *arg)
{
int id = *(int *)arg;
while (1) {
pthread_mutex_lock(&mutex);
while (count == 0) {
printf("consumer %d: buffer is empty, wait...\n", id);
pthread_cond_wait(¬_empty, &mutex);
}
int item = buffer[out];
printf("consumer %d: consume item %d\n", id, item);
out = (out + 1) % BUFFER_SIZE;
count--;
pthread_cond_signal(¬_full);
pthread_mutex_unlock(&mutex);
sleep(2);
}
return NULL;
}
int main(int argc, char *argv[])
{
pthread_t producer_threads[PRODUCER_NUM];
pthread_t consumer_threads[CONSUMER_NUM];
int producer_ids[PRODUCER_NUM];
int consumer_ids[CONSUMER_NUM];
int i;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(¬_full, NULL);
pthread_cond_init(¬_empty, NULL);
for (i = 0; i < PRODUCER_NUM; i++) {
producer_ids[i] = i + 1;
pthread_create(&producer_threads[i], NULL, producer, &producer_ids[i]);
}
for (i = 0; i < CONSUMER_NUM; i++) {
consumer_ids[i] = i + 1;
pthread_create(&consumer_threads[i], NULL, consumer, &consumer_ids[i]);
}
for (i = 0; i < PRODUCER_NUM; i++) {
pthread_join(producer_threads[i], NULL);
}
for (i = 0; i < CONSUMER_NUM; i++) {
pthread_join(consumer_threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(¬_full);
pthread_cond_destroy(¬_empty);
return 0;
}
```
这个程序创建了两个生产者线程和三个消费者线程,它们共享一个缓冲区。当缓冲区已满时,生产者线程等待缓冲区不满的条件变量;当缓冲区为空时,消费者线程等待缓冲区不空的条件变量。生产者线程生产一个随机数并将其放入缓冲区中,消费者线程从缓冲区中取出一个元素并处理它。使用互斥锁来保护缓冲区的读写操作,以避免同时访问缓冲区造成的竞争情况。
阅读全文
相关推荐
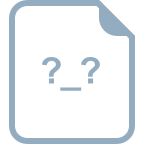
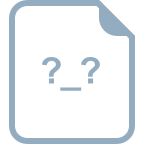
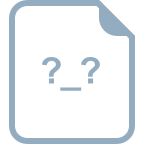















