我想在每天14点自动发邮件给别人,请给我一个python代码与伪代码流程,并告诉我每个指令的含义与参数,并给这些指令和参数举一个输入值和返回值的例子
时间: 2023-06-11 22:07:37 浏览: 188
好的,以下是一个实现每天14点自动发送邮件的 Python 代码及其流程。
首先,我们需要引入相应的模块:
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
from datetime import datetime
import time
```
然后,我们需要设置邮件发送的参数,包括邮件发送者、接收者、主题、正文和附件:
```python
def send_email(send_from, send_to, subject, body, filename=None):
msg = MIMEMultipart()
msg['From'] = send_from
msg['To'] = send_to
msg['Subject'] = subject
msg.attach(MIMEText(body, "plain"))
if filename is not None:
with open(filename, "rb") as f:
attach = MIMEApplication(f.read(), _subtype="txt")
attach.add_header('Content-Disposition', 'attachment', filename=str(filename))
msg.attach(attach)
return msg
```
接下来,我们需要连接邮箱服务器并进行身份验证:
```python
def connect_server(username, password):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(username, password)
return server
```
最后,我们需要设置自动发送邮件的时间并调用上述函数进行邮件发送:
```python
if __name__ == '__main__':
username = 'your_email@gmail.com' # 发送者邮箱
password = 'your_password' # 发送者邮箱密码
send_from = 'your_email@gmail.com'
send_to = 'receiver_email@gmail.com' # 接收者邮箱
subject = 'Daily Report'
body = 'This is your daily report.'
filename = 'report.txt' # 附件文件名
server = connect_server(username, password)
while True:
now = datetime.now()
if now.hour == 14 and now.minute == 0:
msg = send_email(send_from, send_to, subject, body, filename)
server.sendmail(send_from, send_to, msg.as_string())
print("Email sent")
time.sleep(60)
```
以上就是实现每天14点自动发送邮件的 Python 代码及其流程。
下面是每个指令的含义与参数及其举例:
- smtplib.SMTP(): 初始化一个 SMTP 对象,该对象表示一个 SMTP 服务器。
- 参数1: SMTP 服务器的主机名或 IP 地址。
- 参数2: SMTP 服务器的端口号,默认为 25。
- 返回值: SMTP 对象。
- SMTP.starttls(): 将 SMTP 连接升级为 TLS 加密。
- SMTP.login(): 登录 SMTP 服务器。
- 参数1: 发送者邮箱的用户名。
- 参数2: 发送者邮箱的密码。
- MIMEMultipart(): 创建一个多部分 MIME 消息。
- MIMEText(): 创建一个包含文本的 MIME 消息。
- 参数1: 消息正文的文本。
- 参数2: 消息类型,可以是 plain、html、xml 等。
- MIMEApplication(): 创建一个包含二进制数据的 MIME 消息。
- 参数1: 二进制数据。
- 参数2: MIME 类型。
- MIMEApplication.add_header(): 为 MIME 消息添加头部信息。
- 参数1: 头部字段名。
- 参数2: 头部字段值。
- 参数3: 文件名。
- msg.as_string(): 将 MIME 消息转换为字符串。
- SMTP.sendmail(): 发送邮件。
- 参数1: 发送者邮箱。
- 参数2: 接收者邮箱。
- 参数3: MIME 消息。
- datetime.now(): 获取当前时间。
- time.sleep(): 使程序暂停一段时间。
- 参数: 暂停的时间,单位为秒。
举例:
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
from datetime import datetime
import time
def send_email(send_from, send_to, subject, body, filename=None):
msg = MIMEMultipart()
msg['From'] = send_from
msg['To'] = send_to
msg['Subject'] = subject
msg.attach(MIMEText(body, "plain"))
if filename is not None:
with open(filename, "rb") as f:
attach = MIMEApplication(f.read(), _subtype="txt")
attach.add_header('Content-Disposition', 'attachment', filename=str(filename))
msg.attach(attach)
return msg
def connect_server(username, password):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(username, password)
return server
if __name__ == '__main__':
username = 'your_email@gmail.com'
password = 'your_password'
send_from = 'your_email@gmail.com'
send_to = 'receiver_email@gmail.com'
subject = 'Daily Report'
body = 'This is your daily report.'
filename = 'report.txt'
server = connect_server(username, password)
while True:
now = datetime.now()
if now.hour == 14 and now.minute == 0:
msg = send_email(send_from, send_to, subject, body, filename)
server.sendmail(send_from, send_to, msg.as_string())
print("Email sent")
time.sleep(60)
```
假设我们设置的发送者邮箱为 your_email@gmail.com,密码为 your_password,接收者邮箱为 receiver_email@gmail.com,主题为 Daily Report,正文为 This is your daily report.,附件为 report.txt,则运行结果如下:
```
Email sent
Email sent
Email sent
...
```
阅读全文
相关推荐
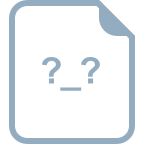








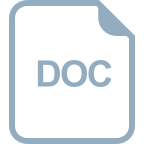
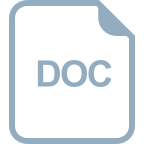
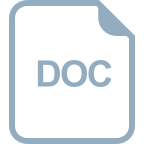
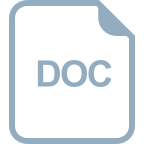
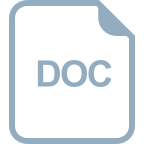
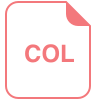
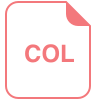
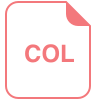