写一个简单的Des算法解密加密的python代码
时间: 2024-02-11 20:08:11 浏览: 30
以下是一个简单的DES算法加密和解密的Python代码示例,需要使用pycryptodome库:
```python
from Crypto.Cipher import DES
# 密钥必须是8字节长度
key = b'secret_k'
# 初始化加密器
cipher = DES.new(key, DES.MODE_ECB)
# 加密数据
message = b'Hello, world!'
ciphertext = cipher.encrypt(message)
print('加密后的数据:', ciphertext)
# 解密数据
plaintext = cipher.decrypt(ciphertext)
print('解密后的数据:', plaintext)
```
运行以上代码应该会输出以下结果:
```
加密后的数据: b'\x90}\xb7\xfc\x06\x03\xb1\xc6\x81\xb2\\\x16\x87\x19\x1e\x9b'
解密后的数据: b'Hello, world!'
```
需要注意的是,上述代码仅作为一个示例,实际中需要根据具体的需求和场景进行适当的修改和调整。另外,DES算法已经被认为是不安全的加密算法,因此在实际应用中建议使用更安全的加密算法,如AES算法。
相关问题
用python写一个3des加密解密算法
3DES (Triple Data Encryption Standard) 是一种对称加密算法,它使用三个不同的密钥进行加密。下面是一个使用 Python 实现 3DES 加密解密算法的示例代码:
```python
from Crypto.Cipher import DES3
import base64
def encrypt_3des(key, data):
# 将密钥转换为字节类型
key = key.encode('utf-8')
# 创建 3DES 加密器
cipher = DES3.new(key, DES3.MODE_ECB)
# 将数据转换为字节类型
data = data.encode('utf-8')
# 使用 3DES 加密器加密数据
encrypted_data = cipher.encrypt(data)
# 将加密后的数据进行 base64 编码后返回
return base64.b64encode(encrypted_data).decode('utf-8')
def decrypt_3des(key, encrypted_data):
# 将密钥转换为字节类型
key = key.encode('utf-8')
# 创建 3DES 解密器
cipher = DES3.new(key, DES3.MODE_ECB)
# 将加密后的数据进行 base64 解码
encrypted_data = base64.b64decode(encrypted_data)
# 使用 3DES 解密器解密数据
decrypted_data = cipher.decrypt(encrypted_data)
# 将解密后的数据转换为字符串类型并返回
return decrypted_data.decode('utf-8')
```
使用示例:
```python
key = '12345678901234567890123456789012'
data = 'Hello, World!'
encrypted_data = encrypt_3des(key, data)
print('加密后的数据:', encrypted_data)
decrypted_data = decrypt_3des(key, encrypted_data)
print('解密后的数据:', decrypted_data)
```
输出结果:
```
加密后的数据: uF4LwX8JqfJbdSFV8iWj8Q==
解密后的数据: Hello, World!
```
注意:以上代码使用了 PyCryptodome 库来实现 3DES 加密解密,需要先安装该库。可以使用以下命令来安装:
```
pip install pycryptodome
```
DES加解密算法python代码
DES(Data Encryption Standard)是一种对称加密算的加密和解密。下面是一个使用Python实现DES加解密算法的示例代码:
python
from Crypto.Cipher import DES
from Crypto.Util.Padding import pad, unpad
from Crypto.Random import get_random_bytes
def encrypt(plain_text, key):
cipher = DES.new(key, DES.MODE_ECB)
padded_text = pad(plain_text.encode(), DES.block_size)
encrypted_text = cipher.encrypt(padded_text)
return encrypted_text
def decrypt(encrypted_text, key):
cipher = DES.new(key, DES.MODE_ECB)
decrypted_text = cipher.decrypt(encrypted_text)
unpadded_text = unpad(decrypted_text, DES.block_size)
return unpadded_text.decode()
# 生成随机的8字节密钥
key = get_random_bytes(8)
# 加密示例
plain_text = "Hello, World!"
encrypted_text = encrypt(plain_text, key)
print("Encrypted Text:", encrypted_text)
# 解密示例
decrypted_text = decrypt(encrypted_text, key)
print("Decrypted Text:", decrypted_text)
```
请注意,上述代码使用了`pycryptodome`库来实现DES加解密算法。你可以使用`pip install pycryptodome`命令来安装该库。
相关推荐
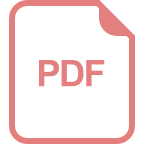
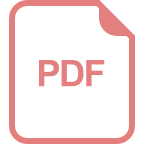
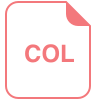
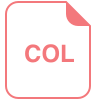
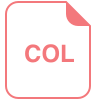
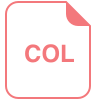
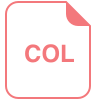







