DES加密解密编程代码python
时间: 2024-09-24 16:28:25 浏览: 72
DES (Data Encryption Standard) 是一种对称加密算法,用于数据的加密和解密。在Python中,我们可以使用`cryptography`库来进行DES操作,这是一个流行的密码学库。下面是一个简单的例子,展示如何使用`cryptography`进行DES加解密:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
# 导入需要的密钥函数
def get_des_key(password):
salt = b'some_salt' # 这里通常会从安全来源获取盐
kdf = PBKDF2HMAC(algorithm=algorithms.HMAC(algorithm=algorithms.SHA256(), backend=default_backend()),
length=16, salt=salt, iterations=100000)
key = kdf.derive(password.encode('utf-8'))
return key
# 加密函数
def encrypt(plaintext, key):
cipher = Cipher(algorithms.DES(key), modes.ECB(), backend=default_backend())
encryptor = cipher.encryptor()
ciphertext = encryptor.update(plaintext.encode('utf-8')) + encryptor.finalize()
return ciphertext
# 解密函数
def decrypt(ciphertext, key):
cipher = Cipher(algorithms.DES(key), modes.ECB(), backend=default_backend())
decryptor = cipher.decryptor()
plaintext = decryptor.update(ciphertext) + decryptor.finalize()
return plaintext.decode('utf-8')
# 示例用法
password = "your_password"
key = get_des_key(password)
plaintext = "Hello, DES!"
ciphertext = encrypt(plaintext, key)
print("Encrypted:", ciphertext)
decrypted_text = decrypt(ciphertext, key)
print("Decrypted:", decrypted_text)
阅读全文
相关推荐



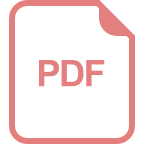
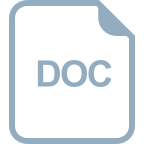
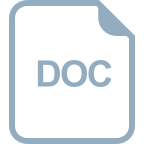










