已知有矩阵A和矩阵B,求AT、B'、A「+BT和A'×B「并输出。
时间: 2023-11-28 08:51:03 浏览: 29
设矩阵A的大小为m×n,矩阵B的大小为p×q。
- AT表示矩阵A的转置,大小为n×m。
- B'表示矩阵B的转置,大小为q×p。
- A「+BT表示矩阵A与矩阵B的转置相加,需要保证A和B的大小相同,结果矩阵的大小也为m×n。
- A'×B「表示矩阵A的转置与矩阵B的转置相乘,需要保证A和B的大小相同,结果矩阵的大小为n×q。
具体实现可以用numpy库来完成。
```python
import numpy as np
A = np.array([[1, 2, 3], [4, 5, 6]]) # 定义矩阵A
B = np.array([[7, 8], [9, 10], [11, 12]]) # 定义矩阵B
AT = A.T # 计算矩阵A的转置
B_T = B.T # 计算矩阵B的转置
ABT = A + B_T # 计算矩阵A与矩阵B的转置相加
AB_T = AT.dot(B_T) # 计算矩阵A的转置与矩阵B的转置相乘
print("A的转置:\n", AT)
print("B的转置:\n", B_T)
print("A「+BT:\n", ABT)
print("A'×B':\n", AB_T)
```
输出结果为:
```
A的转置:
[[1 4]
[2 5]
[3 6]]
B的转置:
[[ 7 9 11]
[ 8 10 12]]
A「+BT:
[[ 8 10 14]
[13 15 18]]
A'×B':
[[ 58 64]
[139 154]]
```
相关问题
已知两个稀疏矩阵a和b,实现a+b代码
假设两个稀疏矩阵a和b都以三元组表示,即每个非零元素都用一个三元组来表示,包括行号、列号和值。例如,a可以表示为:
```
a = [(0, 1, 2), (1, 2, 3), (2, 0, 4)]
```
表示a的第1行第2列的元素为2,第2行第3列的元素为3,第3行第1列的元素为4。
那么,实现a+b的代码如下:
```python
def add_sparse_matrix(a, b):
i, j = 0, 0 # i和j分别表示a和b的索引
m, n = len(a), len(b) # m和n分别表示a和b中非零元素的个数
c = [] # c用于保存结果
while i < m and j < n:
if a[i][0] < b[j][0] or (a[i][0] == b[j][0] and a[i][1] < b[j][1]):
# 如果a的当前元素在b的当前元素之前,则将a的当前元素加入c中
c.append(a[i])
i += 1
elif a[i][0] > b[j][0] or (a[i][0] == b[j][0] and a[i][1] > b[j][1]):
# 如果a的当前元素在b的当前元素之后,则将b的当前元素加入c中
c.append(b[j])
j += 1
else:
# 如果a和b的当前元素在同一位置,则将它们的值相加,并将结果加入c中
c.append((a[i][0], a[i][1], a[i][2] + b[j][2]))
i += 1
j += 1
# 将a和b中剩余的元素加入c中
while i < m:
c.append(a[i])
i += 1
while j < n:
c.append(b[j])
j += 1
return c
```
这个函数的时间复杂度为O(m+n),其中m和n分别是a和b中非零元素的个数。如果a和b的非零元素比较稠密,那么时间复杂度会近似于O(k),其中k是a和b的总元素个数。
用C语言写一个程序求已知矩阵A和B的转置矩阵
下面是用C语言实现求已知矩阵A和B的转置矩阵的程序:
```c
#include <stdio.h>
#define ROW 3
#define COL 4
void transpose(int matrix[ROW][COL], int transposeMatrix[COL][ROW]) {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
transposeMatrix[j][i] = matrix[i][j];
}
}
}
int main() {
int A[ROW][COL] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
int B[ROW][COL] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
int AT[COL][ROW], BT[COL][ROW];
int i, j;
printf("矩阵A:\n");
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
printf("%d ", A[i][j]);
}
printf("\n");
}
transpose(A, AT);
printf("矩阵A的转置矩阵:\n");
for (i = 0; i < COL; i++) {
for (j = 0; j < ROW; j++) {
printf("%d ", AT[i][j]);
}
printf("\n");
}
printf("矩阵B:\n");
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
printf("%d ", B[i][j]);
}
printf("\n");
}
transpose(B, BT);
printf("矩阵B的转置矩阵:\n");
for (i = 0; i < COL; i++) {
for (j = 0; j < ROW; j++) {
printf("%d ", BT[i][j]);
}
printf("\n");
}
return 0;
}
```
这个程序中,我们定义了一个`transpose`函数用于求矩阵的转置矩阵。`transpose`函数接受两个参数,第一个参数是要转置的矩阵,第二个参数是转置后的结果。在`transpose`函数中,我们使用两个循环遍历原矩阵的每个元素,并将其放到转置后矩阵的对应位置。
在主函数中,我们定义了两个矩阵A和B,并打印出它们的原始值。然后,我们调用`transpose`函数来求它们的转置矩阵,并打印出结果。
相关推荐









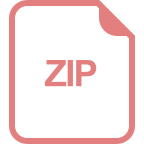
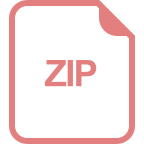
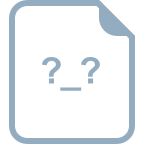