render: (h,params) => { return h('div',{ style: { display: 'flex' } },[ h('i-input',{ props: { value: params.row.price }, on: { input: event => { console.log(event) console.log(params.index) console.log(this.data),中this.data未定义
时间: 2024-02-10 15:13:17 浏览: 122
根据您提供的代码,我看到在render函数中使用了箭头函数,而箭头函数没有自己的this值,它会继承它所在上下文中的this值。因此,在箭头函数中,this指向的是它所在的上下文,而不是Vue组件实例。所以,在这种情况下,this.data未定义,因为它不是指向Vue组件实例的。
要解决这个问题,您可以将箭头函数改为普通函数,并使用bind()方法将this绑定到Vue组件实例。例如,您可以将代码修改为:
```
render: function(h, params) {
return h('div', { style: { display: 'flex' } }, [
h('i-input', {
props: {
value: params.row.price
},
on: {
input: function(event) {
console.log(event);
console.log(params.index);
console.log(this.data);
}.bind(this)
}
})
]);
}
```
这样,this.data就可以正确地指向Vue组件实例了。
相关问题
columns: [ { title: '规格', width: 600, align: 'center', key: 'goodsSpecs' }, { title: '库存', width: 200, align: 'center', key: 'stack', render: (h,params) => { return h('i-input',{ props: { value: params.row.stack }, on: { input: event => { params.row.stack = event.target.value; } } }); } }, { title: '价格', width: 200, align: 'center', key: 'price', render: (h,params) => { return h('div',{ style: { display: 'flex' } },[ h('i-input',{ props: { value: params.row.price }, on: { input: event => { vm.specs[params].price = params.row.price; } } }),h('span','元') ] ); } } ],i-table如何把输入的price绑定给specs的price
在i-table中,要把输入的price绑定给specs的price,可以在输入框的input事件中修改对应的specs对象的price属性。具体实现可以参考以下代码:
```html
<template>
<Table :columns="columns" :data="data"></Table>
</template>
<script>
export default {
data() {
return {
data: [
{ goodsSpecs: '规格1', stack: 10, price: 20 },
{ goodsSpecs: '规格2', stack: 20, price: 30 },
{ goodsSpecs: '规格3', stack: 30, price: 40 }
],
columns: [
{
title: '规格',
width: 600,
align: 'center',
key: 'goodsSpecs'
},
{
title: '库存',
width: 200,
align: 'center',
key: 'stack',
render: (h, params) => {
return h('i-input', {
props: {
value: params.row.stack
},
on: {
input: event => {
params.row.stack = event.target.value;
}
}
});
}
},
{
title: '价格',
width: 200,
align: 'center',
key: 'price',
render: (h, params) => {
return h('div', { style: { display: 'flex' } }, [
h('i-input', {
props: {
value: params.row.price
},
on: {
input: event => {
// 在input事件中修改对应的specs对象的price属性
this.data[params.index].price = event.target.value;
}
}
}),
h('span', '元')
]);
}
}
]
}
}
}
</script>
```
在这个示例中,我们在i-table中定义了一个data数组,其中每个元素代表表格中的一行数据。然后在模板中使用Table组件来定义表格的结构和样式,并通过`:data`属性将数据源绑定到表格上。接着使用Column子组件来定义表格的列,其中第三列的`render`函数返回一个包含一个输入框和一个“元”的div元素。在输入框的`input`事件中,我们修改对应的specs对象的price属性,即可实现输入的price绑定给specs的price的功能。需要注意的是,为了在input事件中访问到当前行和列的信息,我们使用了`params`参数。另外,为了在input事件中访问到this.data数组,我们使用了箭头函数来避免this指向的问题。
设计实现天气预报查询网站,包括查询主页、城市切换、七天天气展示、天气详情页面; 要求: 1.使用Vue作为前端开发框架; 2.使用vue-router实现前端路由的定义及跳转、参数的传递等; 3.使用Axios进行数据请求管理,实现天气数据的获取 vue具体代码
以下是一个简单的天气预报查询网站的Vue代码示例,包括查询主页、城市切换、七天天气展示、天气详情页面。
首先,我们需要在`App.vue`中定义路由和导航栏:
```html
<template>
<div id="app">
<nav>
<router-link to="/">查询</router-link>
</nav>
<router-view />
</div>
</template>
<script>
export default {
name: "App",
components: {},
};
</script>
<style>
nav {
display: flex;
justify-content: center;
background-color: #2196f3;
padding: 10px;
}
nav a {
color: #fff;
margin: 0 10px;
text-decoration: none;
}
nav a.active {
font-weight: bold;
}
</style>
```
然后,在`main.js`中创建路由和Axios实例:
```javascript
import Vue from "vue";
import App from "./App.vue";
import VueRouter from "vue-router";
import axios from "axios";
Vue.use(VueRouter);
Vue.prototype.$http = axios;
const routes = [
{
path: "/",
component: Home,
},
{
path: "/weather/:city",
component: Weather,
},
];
const router = new VueRouter({
mode: "history",
routes,
});
new Vue({
router,
render: (h) => h(App),
}).$mount("#app");
```
其中,`Home`和`Weather`是两个组件,分别对应查询主页和天气详情页面。`Vue.prototype.$http = axios`语句将Axios实例添加到Vue的原型中,以便在组件中使用。
接下来,我们可以实现查询主页组件`Home.vue`:
```html
<template>
<div>
<h1>天气预报查询</h1>
<form @submit.prevent="search">
<label for="city">城市:</label>
<select v-model="city">
<option value="北京">北京</option>
<option value="上海">上海</option>
<option value="广州">广州</option>
<option value="深圳">深圳</option>
<option value="成都">成都</option>
</select>
<button type="submit">查询</button>
</form>
</div>
</template>
<script>
export default {
name: "Home",
data() {
return {
city: "北京",
};
},
methods: {
search() {
this.$router.push(`/weather/${this.city}`);
},
},
};
</script>
```
这里我们使用了一个表单来获取用户输入的城市名称,并在提交表单时将城市名称作为参数传递给`Weather`组件。
然后,我们可以实现天气详情页面组件`Weather.vue`:
```html
<template>
<div>
<h1>{{ city }}天气预报</h1>
<div>
<h2>今天</h2>
<img :src="weather.today.icon" :alt="weather.today.text" />
<p>温度:{{ weather.today.temp }}℃</p>
<p>湿度:{{ weather.today.humidity }}</p>
<p>风向:{{ weather.today.wind }}</p>
</div>
<div v-for="(day, index) in weather.forecast" :key="index">
<h2>{{ day.date }}</h2>
<img :src="day.icon" :alt="day.text" />
<p>温度:{{ day.high }}℃ ~ {{ day.low }}℃</p>
<p>风向:{{ day.wind }}</p>
</div>
</div>
</template>
<script>
export default {
name: "Weather",
data() {
return {
city: "",
weather: null,
};
},
mounted() {
this.city = this.$route.params.city;
this.getWeather();
},
watch: {
"$route.params.city": function () {
this.city = this.$route.params.city;
this.getWeather();
},
},
methods: {
async getWeather() {
const response = await this.$http.get(
`https://api.openweathermap.org/data/2.5/weather?q=${this.city}&appid=YOUR_API_KEY&units=metric&lang=zh_cn`
);
const data = response.data;
this.weather = {
today: {
temp: data.main.temp,
humidity: data.main.humidity,
wind: data.wind.speed,
icon: `https://openweathermap.org/img/w/${data.weather[0].icon}.png`,
text: data.weather[0].description,
},
forecast: [],
};
const forecastResponse = await this.$http.get(
`https://api.openweathermap.org/data/2.5/forecast?q=${this.city}&appid=YOUR_API_KEY&units=metric&lang=zh_cn`
);
const forecastData = forecastResponse.data;
for (let i = 0; i < forecastData.list.length; i += 8) {
const item = forecastData.list[i];
this.weather.forecast.push({
date: item.dt_txt.slice(0, 10),
high: item.main.temp_max,
low: item.main.temp_min,
icon: `https://openweathermap.org/img/w/${item.weather[0].icon}.png`,
text: item.weather[0].description,
wind: item.wind.speed,
});
}
},
},
};
</script>
```
在这里,我们使用了`mounted`钩子和`watch`属性来获取路由参数(城市名称)并获取天气数据。我们使用了`openweathermap`API来获取天气数据,并将其存储在`weather`对象中,然后在模板中使用`v-for`指令来循环渲染未来七天的天气信息。
最后,在`main.js`中,我们需要在Axios实例中添加API密钥:
```javascript
import Vue from "vue";
import App from "./App.vue";
import VueRouter from "vue-router";
import axios from "axios";
Vue.use(VueRouter);
Vue.prototype.$http = axios.create({
baseURL: "https://api.openweathermap.org/data/2.5",
params: {
appid: "YOUR_API_KEY",
units: "metric",
lang: "zh_cn",
},
});
const routes = [
{
path: "/",
component: Home,
},
{
path: "/weather/:city",
component: Weather,
},
];
const router = new VueRouter({
mode: "history",
routes,
});
new Vue({
router,
render: (h) => h(App),
}).$mount("#app");
```
在这里,我们使用了`axios.create`方法来创建一个新的Axios实例,并在其参数中添加了`baseURL`和`params`,以便在每个请求中自动添加API密钥和其他参数。
这是一个简单的天气预报查询网站的Vue代码示例,你可以根据实际需求进行修改和完善。
阅读全文
相关推荐
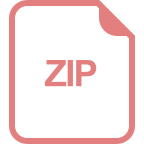
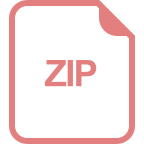
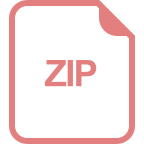
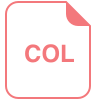
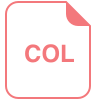
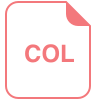
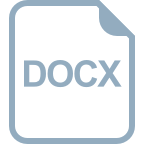
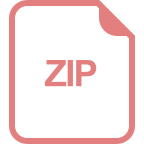
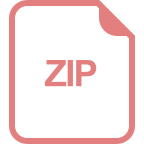