设置一个arduino密码锁
时间: 2023-11-15 09:04:29 浏览: 144
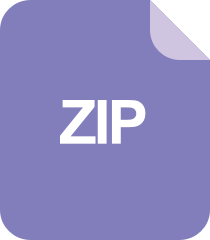
利用Arduino和app inventor制作一个简单密码锁

要设置一个基于Arduino的密码锁,您需要以下部件:
- Arduino Uno板
- 4x4矩阵键盘
- 16x2字符LCD显示器
- 电位器
- 电位器旋钮
- 9V电池
步骤:
1.将4x4矩阵键盘连接到Arduino Uno板的数字引脚,以便使用数字引脚读取钥匙按下的值。
2.将16x2字符LCD显示器连接到Arduino Uno板的数字引脚和电源引脚,以便显示消息和密码。
3.将电位器安装在Arduino Uno板上,并将电位器旋钮连接到LCD显示器上,以便调整对比度。
4.编写Arduino程序,以便:
- 读取按下的钥匙值
- 显示消息和密码
- 比较输入的密码与预定义密码
- 控制锁定/解锁的电路
5.将9V电池连接到Arduino Uno板上,以便在没有电源插座的情况下,提供电源。
下面是一个示例代码,您可以根据需要进行修改:
```
#include <LiquidCrystal.h> //引入LCD库
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); //定义LCD引脚
const int ROWS = 4; //定义行数
const int COLS = 4; //定义列数
char keys[ROWS][COLS] = { //定义按键值
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
int rowPins[ROWS] = {9, 8, 7, 6}; //定义行引脚
int colPins[COLS] = {5, 4, 3, 2}; //定义列引脚
int password[] = {1, 2, 3, 4}; //定义密码
int enteredPassword[] = {0, 0, 0, 0}; //定义输入的密码
int cursorPosition = 0; //定义光标位置
bool unlocked = false; //定义锁状态
void setup() {
lcd.begin(16, 2); //初始化LCD
pinMode(13, OUTPUT); //定义锁电路引脚
digitalWrite(13, LOW); //锁电路关闭
}
void loop() {
if (!unlocked) { //如果锁未解锁,则输入密码
char key = getKey(); //获取按键值
if (key != '\0') { //如果有按键被按下
enteredPassword[cursorPosition] = key - '0'; //更新输入的密码
cursorPosition++; //移动光标
lcd.clear(); //清除LCD屏幕
lcd.print("Enter password:"); //显示提示消息
lcd.setCursor(0, 1); //移动光标到第二行
for (int i = 0; i < 4; i++) { //显示输入的密码
if (i < cursorPosition) {
lcd.print(enteredPassword[i]);
} else {
lcd.print("-");
}
}
if (cursorPosition == 4) { //如果输入的密码长度为4,则比较密码
bool correctPassword = true;
for (int i = 0; i < 4; i++) {
if (enteredPassword[i] != password[i]) {
correctPassword = false;
break;
}
}
if (correctPassword) { //如果密码正确,则解锁
lcd.clear();
lcd.print("Unlocked!");
digitalWrite(13, HIGH);
unlocked = true;
} else { //否则重新输入密码
lcd.clear();
lcd.print("Wrong password!");
delay(1000);
lcd.clear();
lcd.print("Enter password:");
cursorPosition = 0;
}
}
}
} else { //如果锁已解锁,则等待重新锁定
char key = getKey();
if (key == '*') { //如果按下星号键,则重新锁定
digitalWrite(13, LOW);
unlocked = false;
cursorPosition = 0;
for (int i = 0; i < 4; i++) {
enteredPassword[i] = 0;
}
lcd.clear();
lcd.print("Enter password:");
}
}
}
char getKey() { //获取按键值
for (int col = 0; col < COLS; col++) {
pinMode(colPins[col], OUTPUT);
digitalWrite(colPins[col], LOW);
for (int row = 0; row < ROWS; row++) {
pinMode(rowPins[row], INPUT_PULLUP);
if (digitalRead(rowPins[row]) == LOW) {
delay(50);
if (digitalRead(rowPins[row]) == LOW) {
return keys[row][col];
}
}
}
pinMode(rowPins[col], OUTPUT);
digitalWrite(rowPins[col], HIGH);
}
return '\0';
}
```
请注意,这只是一个示例代码,您需要根据需要进行修改,例如更改密码,更改提示消息等等。
阅读全文
相关推荐
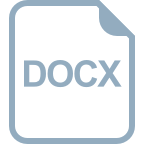
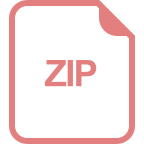













