.net core web api 中使用了 JwtBearer 生成 token,如何把 token 放在 cookie 中
时间: 2024-10-19 07:16:48 浏览: 39
在.NET Core Web API中,如果你想要将JWT(JSON Web Token)存储在HTTP Cookie中,你需要使用Microsoft.AspNetCore.Authentication.JwtBearer库,同时配合CookieAuthentication选项。以下是一个简单的步骤:
1. 首先,在Startup.cs文件中添加对JwtBearer和Cookie中间件的支持。在`ConfigureServices`方法中添加JWT验证服务,并在`Configure`方法中添加Cookie认证:
```csharp
public void ConfigureServices(IServiceCollection services)
{
// 添加 JWT 验证服务
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuerSigningKey = true,
ValidateIssuer = true,
ValidateAudience = true,
// ... (其他验证设置)
};
});
// 添加 Cookie 认证服务
services.AddCookies();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// ... 其他配置
// 将 JWT Bearer 中间件放置在 Cookie 中间件之前
app.UseRouting();
app.UseAuthentication(); // 这会让 JWT Bearer 作用于所有请求
app.UseAuthorization();
// 使用 Cookie Authentication 中间件,将 JWT 的身份信息放入cookie
app.UseCookieAuthentication(new CookieAuthenticationOptions
{
AutomaticAuthenticate = true,
AutomaticChallenge = true,
LoginPath = "/Account/Login", // 登录路径
LogoutPath = "/Account/Logout",
TicketDataFormat = new CustomJwtFormat(),
});
}
```
这里的关键在于`TicketDataFormat`属性,需要自定义一个`CustomJwtFormat`类,以便将JWT内容转换成Cookie可以携带的数据格式:
```csharp
public class CustomJwtFormat : DataFormatTicketSerializer
{
protected override string ConvertTicketToString(Ticket data)
{
var jwtToken = data.Properties.Items[typeof(JwtSecurityToken).Name] as JwtSecurityToken;
return jwtToken != null ? jwtToken.ToString() : base.ConvertTicketToString(data);
}
protected override Ticket ConvertStringToTicket(string text)
{
var jwtToken = Jwt.Parse(text);
return new ClaimsPrincipal(jwtToken.Claims).CreateIdentity(data);
}
}
```
现在,当你登录成功并获取到JWT token后,它会被自动封装到名为`Auth`或默认名称的HTTP Cookie中。
阅读全文
相关推荐
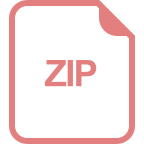
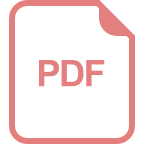

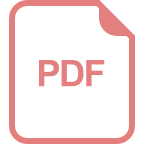
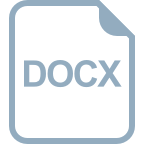
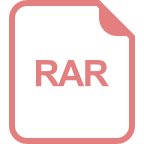
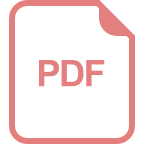
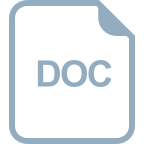
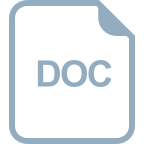
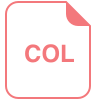
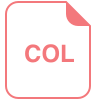
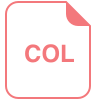
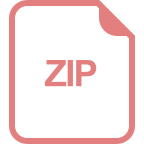
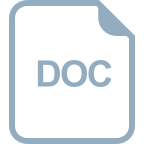

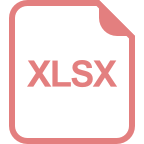
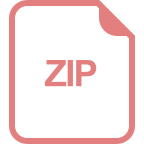
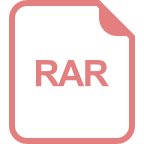
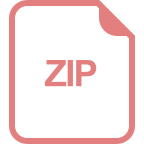