java 有两个key的map吗
时间: 2024-03-20 19:45:00 浏览: 11
Java 标准库中的 Map 接口只支持一对一映射,也就是说,每个键只能对应一个值。因此,Java 标准库中没有原生支持两个 key 的 Map 实现。
不过,可以通过一些方法实现类似两个 key 的 Map 功能。其中一种方法是使用一个元组(Tuple)来作为 Map 的 key,元组中包含两个原本作为 key 的对象。例如,可以使用 Apache Commons Lang 库中的 Pair 类来实现:
```java
import org.apache.commons.lang3.tuple.Pair;
Map<Pair<String, String>, Integer> map = new HashMap<>();
map.put(Pair.of("key1", "key2"), 1);
map.put(Pair.of("key3", "key4"), 2);
System.out.println(map.get(Pair.of("key1", "key2"))); // output: 1
```
另一种方法是使用自定义对象作为 Map 的 key,该对象中包含两个 key 对象,并且实现了 hashCode() 和 equals() 方法。例如:
```java
class TwoKeys {
private final String key1;
private final String key2;
public TwoKeys(String key1, String key2) {
this.key1 = key1;
this.key2 = key2;
}
public String getKey1() {
return key1;
}
public String getKey2() {
return key2;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TwoKeys twoKeys = (TwoKeys) o;
return Objects.equals(key1, twoKeys.key1) && Objects.equals(key2, twoKeys.key2);
}
@Override
public int hashCode() {
return Objects.hash(key1, key2);
}
}
Map<TwoKeys, Integer> map = new HashMap<>();
map.put(new TwoKeys("key1", "key2"), 1);
map.put(new TwoKeys("key3", "key4"), 2);
System.out.println(map.get(new TwoKeys("key1", "key2"))); // output: 1
```
这里我们使用了一个自定义的 TwoKeys 类,它包含了两个 key 对象,实现了 equals() 和 hashCode() 方法。这样就可以将 TwoKeys 对象作为 Map 的 key 使用,实现了类似两个 key 的 Map 功能。
相关推荐
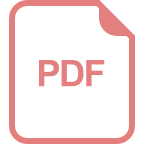
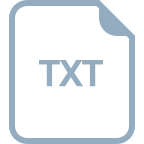














