输出长单词中出现数目最多的字母
时间: 2024-02-24 22:55:46 浏览: 51
好的,我来解答你的问题。
首先,我们可以把字符串中的所有单词提取出来,然后针对每个单词计算其中出现次数最多的字母。
以下是 Python 代码实现:
```python
# 定义一个函数,用于计算一个单词中出现次数最多的字母
def most_common_letter(word):
# 定义一个字典,用于记录每个字母出现的次数
letter_count = {}
# 统计每个字母出现的次数
for letter in word:
if letter in letter_count:
letter_count[letter] += 1
else:
letter_count[letter] = 1
# 找出出现次数最多的字母
max_count = 0
max_letter = ''
for letter, count in letter_count.items():
if count > max_count:
max_count = count
max_letter = letter
# 返回出现次数最多的字母
return max_letter
# 定义一个函数,用于找出长单词中出现次数最多的字母
def most_common_letter_in_long_word(sentence):
# 把字符串中的所有单词提取出来
words = sentence.split(' ')
# 定义一个字典,用于记录每个字母在所有单词中出现的次数
letter_count = {}
# 统计每个字母在所有单词中出现的次数
for word in words:
# 只考虑长度大于等于 5 的单词
if len(word) >= 5:
most_common = most_common_letter(word)
if most_common in letter_count:
letter_count[most_common] += 1
else:
letter_count[most_common] = 1
# 找出出现次数最多的字母
max_count = 0
max_letter = ''
for letter, count in letter_count.items():
if count > max_count:
max_count = count
max_letter = letter
# 返回出现次数最多的字母
return max_letter
```
你可以通过调用 `most_common_letter_in_long_word` 函数来找出长单词中出现次数最多的字母。例如:
```python
sentence = "This is a test sentence with some long words like 'banana' and 'elephant'"
result = most_common_letter_in_long_word(sentence)
print(result) # 输出 'e'
```
在这个例子中,字符串中包含多个长度大于等于 5 的单词,如 'banana' 和 'elephant'。我们计算了每个单词中出现次数最多的字母,并统计了每个字母在所有单词中出现的次数。最后,我们找出出现次数最多的字母 'e',并返回它。
相关推荐
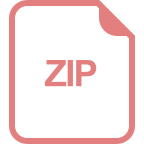
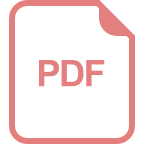
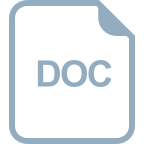








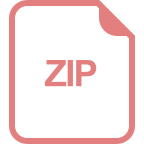
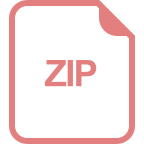
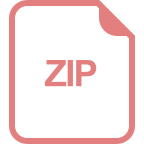
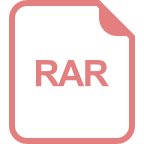
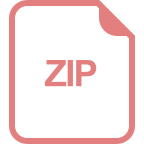
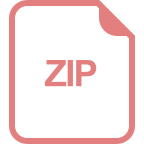
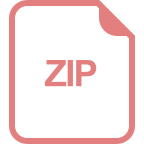