python 批量灰度化
时间: 2023-10-13 10:16:54 浏览: 31
以下是一种Python批量灰度化图像的方法:
``` python
import os
from PIL import Image
# 定义灰度化函数
def convert_to_gray_scale(image_path):
img = Image.open(image_path).convert('L')
img.save(image_path)
# 获取当前工作目录下所有jpg格式的图片
images = [f for f in os.listdir('.') if f.endswith('.jpg')]
# 灰度化所有图片
for img in images:
convert_to_gray_scale(img)
```
首先,需要导入`os`和`PIL`模块。`os`模块用于获取当前工作目录下的文件列表,`PIL`模块用于图像处理。
接下来,定义一个名为`convert_to_gray_scale()`的函数,该函数将指定路径的图像转换为灰度图像。`Image.open()`方法用于打开指定路径的图像,`.convert('L')`方法将图像转换为灰度图像,最后使用`.save()`方法保存更改后的图像。
然后,使用列表推导式获取所有以`.jpg`结尾的文件列表。
最后,使用`for`循环遍历所有图像,将它们传递给`convert_to_gray_scale()`函数以灰度化它们。
相关问题
python批量将图片灰度化
你可以使用Python的PIL库(Pillow)来批量将图片灰度化。下面是一个简单的示例代码:
```python
from PIL import Image
import os
def convert_to_grayscale(image_path):
# 打开图片
image = Image.open(image_path)
# 将图片转换为灰度图像
gray_image = image.convert("L")
# 保存灰度图像
gray_image.save(image_path)
# 遍历指定目录下的所有图片文件
def batch_convert_to_grayscale(directory):
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
image_path = os.path.join(directory, filename)
convert_to_grayscale(image_path)
# 调用函数进行批量灰度化
batch_convert_to_grayscale("your_directory_path")
```
将代码中的"your_directory_path"替换为包含图片文件的目录路径。这段代码将会遍历该目录下的所有图片文件(.jpg和.png),并将它们转换为灰度图像,覆盖原始文件。
请确保在运行代码之前安装了Pillow库,可以使用以下命令进行安装:
```
pip install Pillow
```
希望对你有所帮助!如有其他问题,请随时提问。
python图像批量灰度
实现图像批量灰度化的步骤如下:
1. 导入OpenCV库和os库。OpenCV用于图像处理,os库用于文件操作。
```python
import cv2
import os
```
2. 定义待处理图像文件夹路径和输出图像文件夹路径。
```python
input_folder = "input/"
output_folder = "output/"
```
3. 遍历待处理图像文件夹中的所有图像文件,并对每个文件进行灰度化处理。
```python
for filename in os.listdir(input_folder):
# 读取原始图像
img = cv2.imread(input_folder + filename)
# 将图像转换为灰度图像
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 保存灰度图像
cv2.imwrite(output_folder + filename, gray_img)
```
4. 完整代码如下:
```python
import cv2
import os
input_folder = "input/"
output_folder = "output/"
for filename in os.listdir(input_folder):
# 读取原始图像
img = cv2.imread(input_folder + filename)
# 将图像转换为灰度图像
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 保存灰度图像
cv2.imwrite(output_folder + filename, gray_img)
```
注意:上述代码只处理了常见的BGR格式图像。如果待处理的图像文件夹中还包含其他格式的图像,需要根据实际情况进行修改。
相关推荐
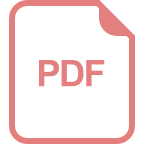
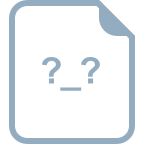












