c语言insert函数怎么编写
时间: 2024-01-20 13:03:14 浏览: 48
C语言中的insert函数可以分为两种,一种是用于向数组中插入元素,另一种是用于向链表中插入节点。
1. 插入元素到数组中
插入元素到数组中,通常需要指定插入位置、插入值以及数组的长度等参数。下面是一个简单的实现:
```c
void insert(int arr[], int len, int pos, int val) {
if (pos < 0 || pos > len) {
printf("Invalid position.\n");
return;
}
for (int i = len - 1; i >= pos; i--) {
arr[i+1] = arr[i];
}
arr[pos] = val;
}
```
其中,arr是数组的指针,len是数组的长度,pos是插入位置,val是要插入的值。
2. 插入节点到链表中
插入节点到链表中,需要指定插入位置、插入节点以及链表头指针等参数。下面是一个简单的实现:
```c
typedef struct node {
int data;
struct node *next;
} Node;
void insert(Node **head, int pos, int val) {
Node *new_node = (Node*) malloc(sizeof(Node));
new_node->data = val;
if (pos == 0) {
new_node->next = *head;
*head = new_node;
} else {
Node *cur_node = *head;
for (int i = 0; i < pos - 1 && cur_node != NULL; i++) {
cur_node = cur_node->next;
}
if (cur_node == NULL) {
printf("Invalid position.\n");
return;
}
new_node->next = cur_node->next;
cur_node->next = new_node;
}
}
```
其中,head是链表头指针,pos是插入位置,val是要插入的值。
相关推荐
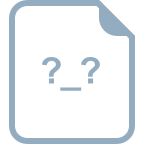
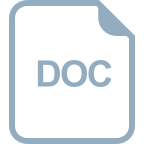
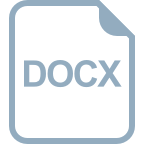
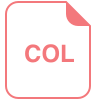













