#include <iostream> using namespace std; //获取参数方式 cin //int x =0; //cin >> x; //结果输出使用 cout //cout<<"1"; // 请在此添加你的代码 /********** Begin ********/ int main() { int n; cin>>n ; double *scores = new double[n]; for (int i=0;i<n;i++) { cin>>scores[i]; } int id; cin>>id; if(id<=1||id>n) { cout<<"Error"<<endl; } else { cout<< scores[id-1]<<endl; } delete[] scores; return 0; } 解释这段代码
时间: 2024-02-17 12:15:24 浏览: 23
这段代码是一个简单的程序,用于从用户输入中读取一些分数,然后输出特定位置的分数值。
程序开始使用 `#include` 命令引入了头文件 iostream,这个头文件包含了标准输入输出流的定义。接着使用 `using namespace std` 命令将 std 命名空间中的名称引入到当前的命名空间中,使得在程序中可以直接使用 std 命名空间中的名称而不需要添加 std:: 前缀。
接下来是程序的主函数 `main()`,其中定义了一个变量 `n` 用于存储输入的分数数量。使用 `new` 关键字动态地为 scores 数组分配内存空间,该数组的大小由用户输入的 n 决定。然后使用 `for` 循环读取用户输入的分数值,并存储到 scores 数组中。
程序接着读取一个整数 `id`,并进行条件判断。如果 id 的值小于等于 1 或者大于 n,则输出 "Error";否则,输出 scores 数组中第 id-1 个元素的值。
最后使用 `delete[]` 关键字释放 scores 数组占用的内存空间,并返回 0 退出程序。
相关问题
#include <iostream> using namespace std; int main() {
这是一个C++程序,它的功能是输出一个n*n的矩阵,其中每个元素都是从n*n开始递减1的数字。下面是代码的解释:
```c++
#include <iostream> // 引入iostream库,用于输入输出
#include <iomanip> // 引入iomanip库,用于格式化输出
using namespace std; // 使用std命名空间
int main() { // 主函数
int n,i,k=0; // 定义整型变量n、、k,并初始化k为0
cin>>n; // 从标准输入中读取n的值
for(i=n*n;i>=1;i--) // 循环n*n次,从n*n开始递减1
{
cout<<setw(5)<<i; // 输出当前数字,setw(5)用于设置输出宽度为5
k++; // k加1
if(k%n==0) // 如果k是n的倍数,说明当前行已经输出完毕
cout<<endl; // 输出换行符
}
cout<<endl; // 输出一个额外的换行符
return 0; // 返回0,表示程序正常结束
}
```
改进代码#include<iostream> using namespace std; int main() { int n; cin >> n; cout << n << endl; return 0; }
您可以尝试使用更加规范的代码风格,例如:
```c++
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int main() {
int n;
cin >> n;
cout << n << endl;
return 0;
}
```
这样可以使代码更加易读,也符合 C++ 的风格规范。另外,如果您想要让程序更具健壮性,可以考虑增加对输入的检查,例如:
```c++
#include <iostream>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
int main() {
int n;
while (!(cin >> n)) { // 如果输入不是一个整数,则重新输入
cin.clear(); // 清除错误标志
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // 忽略缓冲区中的数据,直到遇到换行符
cout << "Invalid input, please try again." << endl;
}
cout << n << endl;
return 0;
}
```
这样可以避免用户输入非法数据导致程序崩溃的问题。
相关推荐
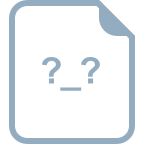
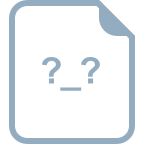
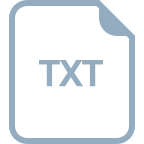













