SpringBoot 将数据库数据导出成txt 并实现下载功能
时间: 2023-11-22 14:52:53 浏览: 169
要将数据库数据导出成txt并实现下载功能,可以按照以下步骤进行:
1. 在Spring Boot项目中添加相关依赖,如Apache POI和Apache Commons IO等。
2. 创建一个Controller,用于接收请求并响应下载文件。
```java
@RestController
public class ExportController {
@Autowired
private ExportService exportService;
@GetMapping("/export")
public void exportData(HttpServletResponse response) throws Exception {
// 文件名
String fileName = "data.txt";
// 文件内容
String content = exportService.exportData();
// 设置响应头信息
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + fileName);
// 写入文件流
ServletOutputStream out = response.getOutputStream();
out.write(content.getBytes());
out.flush();
out.close();
}
}
```
3. 创建一个Service,用于从数据库中查询数据并导出为txt格式。
```java
@Service
public class ExportService {
@Autowired
private JdbcTemplate jdbcTemplate;
public String exportData() {
// 查询数据
List<Map<String, Object>> dataList = jdbcTemplate.queryForList("SELECT * FROM user");
// 导出为txt格式
StringBuilder sb = new StringBuilder();
for (Map<String, Object> data : dataList) {
sb.append(data.get("id")).append("\t")
.append(data.get("name")).append("\t")
.append(data.get("age")).append("\r\n");
}
return sb.toString();
}
}
```
4. 访问http://localhost:8080/export即可下载文件。
注意:以上代码仅为示例,实际应用中需要根据具体情况进行修改。另外,如果数据量较大,建议使用分页查询和流式写入方式,避免内存溢出。
阅读全文
相关推荐
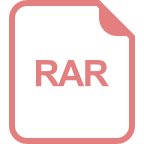

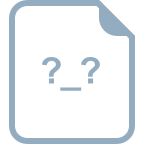
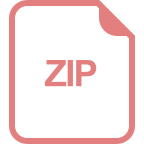
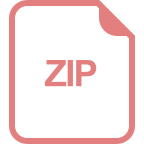
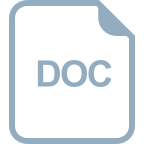
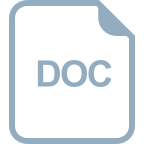


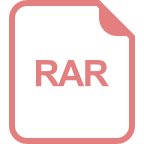
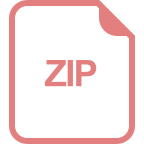
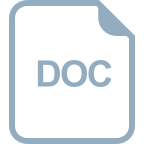
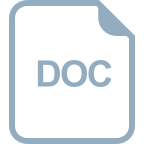
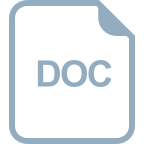
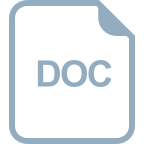
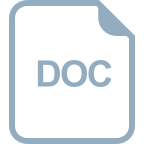
