用C语言编写 任务:编写程序从键盘输入5个学生的信息,包括学生学号、姓名、性别、出生年月及成绩,用结构体数组保存学生的信息,要求用函数实现结构体数组的输入输出及求成绩最高学生的序号。
时间: 2024-03-05 18:50:15 浏览: 54
好的,以下是用C语言编写的程序,可以实现你的需求:
```c
#include <stdio.h>
#include <string.h>
struct student {
char id[20];
char name[20];
char sex;
char birth[20];
float score;
};
void input(struct student stu[], int n);
void output(struct student stu[], int n);
int max_score(struct student stu[], int n);
int main() {
struct student stu[5];
int max_index;
// 输入学生信息
input(stu, 5);
// 输出学生信息
output(stu, 5);
// 求成绩最高学生的序号
max_index = max_score(stu, 5);
printf("成绩最高的学生是:%s,序号为%d\n", stu[max_index].name, max_index + 1);
return 0;
}
void input(struct student stu[], int n) {
int i;
for (i = 0; i < n; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%s", stu[i].id);
printf("请输入第%d个学生的姓名:", i + 1);
scanf("%s", stu[i].name);
printf("请输入第%d个学生的性别(M/F):", i + 1);
scanf(" %c", &stu[i].sex);
printf("请输入第%d个学生的出生年月:", i + 1);
scanf("%s", stu[i].birth);
printf("请输入第%d个学生的成绩:", i + 1);
scanf("%f", &stu[i].score);
}
}
void output(struct student stu[], int n) {
int i;
printf("学号\t姓名\t性别\t出生年月\t成绩\n");
for (i = 0; i < n; i++) {
printf("%s\t%s\t%c\t%s\t\t%.2f\n", stu[i].id, stu[i].name, stu[i].sex, stu[i].birth, stu[i].score);
}
}
int max_score(struct student stu[], int n) {
int i, max_index = 0;
float max_score = stu[0].score;
for (i = 1; i < n; i++) {
if (stu[i].score > max_score) {
max_score = stu[i].score;
max_index = i;
}
}
return max_index;
}
```
程序中定义了一个结构体student,包含了学生的学号、姓名、性别、出生年月及成绩。然后通过input函数从键盘输入5个学生的信息,用结构体数组保存。通过output函数输出学生的信息。通过max_score函数求出成绩最高的学生的序号。在输入学生的性别时,同样要在%c前面加一个空格,以避免scanf函数读取上一次输入的回车符。
相关推荐
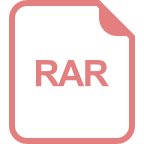
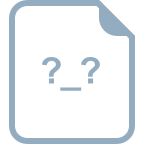
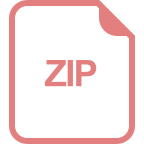
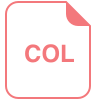
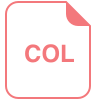
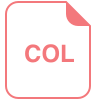
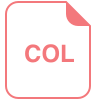
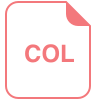





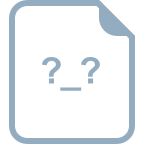
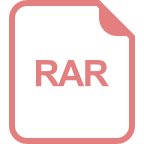
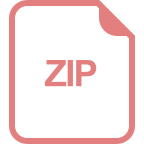
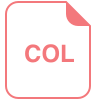
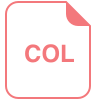