pyqt 以qframe为父类写一个类,并在主界面按坐标放置
时间: 2023-09-13 10:05:24 浏览: 48
### 回答1:
可以使用PyQt的QFrame类作为父类创建自定义类,并在主界面中使用setGeometry()方法按照坐标放置。
示例代码:
```
from PyQt5.QtWidgets import QFrame, QApplication
class MyFrame(QFrame):
def __init__(self):
super().__init__()
self.setGeometry(100, 100, 200, 200)
if __name__ == '__main__':
app = QApplication([])
frame = MyFrame()
frame.show()
app.exec_()
```
这将创建一个宽200像素,高200像素,左上角坐标为(100,100)的QFrame。
### 回答2:
在使用PyQt编写一个以QFrame为父类的类,并在主界面按坐标放置的过程中,您可以按照以下步骤进行操作:
1. 首先,导入PyQt模块和必要的类:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QFrame
from PyQt5.QtCore import Qt
```
2. 创建一个新类,以QFrame为父类,并定义该类的一些属性和方法:
```python
class MyFrame(QFrame):
def __init__(self):
super().__init__()
self.setFrameStyle(QFrame.Box) # 设置边框样式
self.setStyleSheet("background-color: blue;") # 设置背景颜色
self.setGeometry(100, 100, 200, 200) # 设置初始位置和大小
```
3. 创建一个主窗口类:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Main Window") # 设置窗口标题
self.setGeometry(300, 300, 500, 500) # 设置窗口位置和大小
self.initUI()
def initUI(self):
frame = MyFrame() # 创建一个自定义的QFrame对象
self.setCentralWidget(frame) # 设置主窗口的中心控件为自定义的QFrame对象
```
4. 创建一个QApplication实例,并在该实例中显示主窗口:
```python
if __name__ == '__main__':
app = QApplication([])
main_window = MainWindow()
main_window.show()
app.exec_()
```
通过以上步骤,您可以通过定义自己的类继承QFrame类,并在主窗口中按坐标放置该自定义的QFrame实例。需要注意的是,QFrame的坐标是相对于父容器的,所以您可以在MyFrame类的构造函数中使用`self.setGeometry(x, y, width, height)`函数设置自定义QFrame的位置和大小。
### 回答3:
PyQt是一个功能强大的Python GUI库,它可以用来创建交互式的图形用户界面。其中的QFrame类是一个用于显示和布局控件的容器,我们可以利用这个类来创建自定义的界面。
下面是以QFrame为父类写一个类,并在主界面按坐标放置的示例:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QFrame
class CustomFrame(QFrame):
def __init__(self, parent=None):
super().__init__(parent)
self.setGeometry(100, 100, 200, 200) # 设置这个自定义frame的位置和大小
self.setStyleSheet("background-color: red;") # 设置背景颜色为红色
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setGeometry(200, 200, 500, 500) # 设置主窗口的位置和大小
self.setWindowTitle("Main Window")
custom_frame = CustomFrame(self) # 创建一个自定义的frame,并将主窗口作为父容器
self.setCentralWidget(custom_frame) # 将自定义frame设置为主窗口的中心控件
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例中,我们定义了一个CustomFrame类,继承自QFrame类。在CustomFrame的构造函数中,我们设置了自定义frame的位置和大小,以及背景颜色。然后我们创建了一个MainWindow类,继承自QMainWindow类,在主窗口的构造函数中,我们设置了主窗口的位置和大小,并创建了一个CustomFrame实例。最后,我们将这个CustomFrame实例设置为主窗口的中心控件。
通过这样的布局方式,我们可以在主界面按坐标放置自定义的QFrame类,从而创建出自己想要的界面布局。
相关推荐
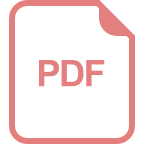














