decimal如何设置全局小数保留位数
时间: 2024-02-21 18:00:53 浏览: 34
你可以使用 `decimal.getcontext()` 方法来获取全局的上下文信息,并在上下文信息中设置小数保留位数。例如,如果要将全局小数保留位数设置为2,可以使用以下代码:
```
import decimal
# 获取全局上下文信息
context = decimal.getcontext()
# 设置小数保留位数为2
context.prec = 2
```
这样,在使用 decimal 进行计算时,所有的结果都会按照小数保留位数为2来进行四舍五入。如果需要单独控制某个运算的保留位数,可以在运算中使用 `quantize()` 方法来设置。
相关问题
设置C#float保留小数位数
要设置C#中的float类型保留小数位数,可以使用以下代码:
```csharp
float number = 3.14159f; // 原始的float数值
float roundedNumber = (float)Math.Round(number, 2); // 保留两位小数
Console.WriteLine(roundedNumber); // 输出结果: 3.14
```
在上述代码中,我们使用`Math.Round()`方法将浮点数四舍五入到指定的小数位数。在这个例子中,我们将浮点数`number`四舍五入到两位小数,并将结果存储在`roundedNumber`变量中。最后,我们通过`Console.WriteLine()`方法将结果打印到控制台。
请注意,由于float类型的精度限制,可能会出现舍入误差。如果需要更高的精度,可以考虑使用decimal类型。
mysql将decimal不保留小数
DECIMAL数据类型在MySQL中用于存储精确的小数值。通过指定整数部分和小数部分的位数,可以决定DECIMAL列的精度。如果未指定小数部分的位数,则默认为0,即DECIMAL(10,0)。DECIMAL列可以保留小数点后的位数,无论是固定的还是浮动的。
如果你希望在MySQL中将DECIMAL不保留小数,你可以使用ROUND()函数将其舍入到指定的位数。例如,如果你想将DECIMAL列的值舍入到0位小数点,你可以使用以下语句:
SELECT ROUND(column_name, 0) FROM table_name;
这将返回不保留小数的整数值。
相关推荐
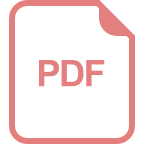
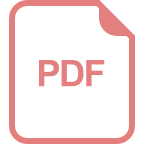
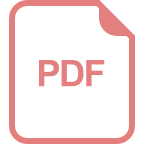











