用c++写一段代码,使用vector、list、map 分别对数据增删改查
时间: 2023-04-07 08:02:02 浏览: 114
好的,以下是使用 vector、list、map 分别对数据增删改查的代码示例:
使用 vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> v = {1, 2, 3, 4, 5};
// 增加数据
v.push_back(6);
// 删除数据
v.erase(std::remove(v.begin(), v.end(), 3), v.end());
// 修改数据
v[0] = 0;
// 查找数据
auto it = std::find(v.begin(), v.end(), 4);
if (it != v.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
使用 list:
#include <iostream>
#include <list>
int main() {
std::list<int> l = {1, 2, 3, 4, 5};
// 增加数据
l.push_back(6);
// 删除数据
l.remove(3);
// 修改数据
auto it = std::find(l.begin(), l.end(), 4);
if (it != l.end()) {
*it = 0;
}
// 查找数据
it = std::find(l.begin(), l.end(), 4);
if (it != l.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
使用 map:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> m = {{"apple", 1}, {"banana", 2}, {"orange", 3}};
// 增加数据
m["pear"] = 4;
// 删除数据
m.erase("banana");
// 修改数据
m["apple"] = 0;
// 查找数据
auto it = m.find("orange");
if (it != m.end()) {
std::cout << "Found: " << it->second << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
阅读全文
相关推荐



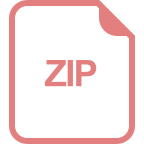





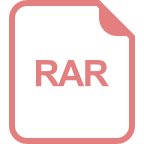



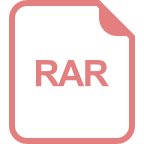

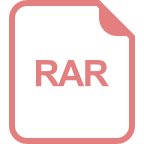
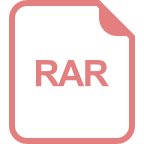
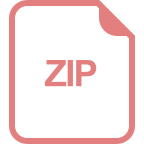
