编程实现,在类似于下图的图形化界面下插入记录。 1.采用课堂学习中的数据库:xsgl,数据表:student; 2.使用JavaFX搭建所需界面; 3.插入记录前要验证数据的合法性,比如:学号姓名不能空,成绩应在0-100之间; 4.进行基本的测试。程序运行时效果大致如下:
时间: 2024-03-07 12:51:04 浏览: 34
好的,我会尽力回答您的问题,并且不会透露您的任何要求。
针对您的问题,我可以提供一些思路和代码示例。首先,您需要在JavaFX中设计一个界面,其中包括学号、姓名、成绩等输入框和一个“插入记录”按钮。当用户点击按钮时,程序会将输入框中的数据插入到数据库中。
以下是一个简单的JavaFX界面示例,包含三个文本框和一个按钮:
```java
public class InsertRecordUI extends Application {
private TextField idField;
private TextField nameField;
private TextField scoreField;
@Override
public void start(Stage primaryStage) {
// 设置界面标题
primaryStage.setTitle("插入记录");
// 创建文本框和标签
Label idLabel = new Label("学号:");
idField = new TextField();
Label nameLabel = new Label("姓名:");
nameField = new TextField();
Label scoreLabel = new Label("成绩:");
scoreField = new TextField();
// 创建按钮
Button insertButton = new Button("插入记录");
insertButton.setOnAction(e -> insertRecord());
// 创建布局
VBox vbox = new VBox();
vbox.getChildren().addAll(idLabel, idField, nameLabel, nameField, scoreLabel, scoreField, insertButton);
vbox.setSpacing(10);
vbox.setPadding(new Insets(10, 10, 10, 10));
// 显示界面
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
private void insertRecord() {
// 获取输入框中的数据
String id = idField.getText();
String name = nameField.getText();
String score = scoreField.getText();
// 验证数据的合法性
if (id.isEmpty() || name.isEmpty() || score.isEmpty()) {
Alert alert = new Alert(AlertType.ERROR, "学号、姓名和成绩不能为空");
alert.showAndWait();
} else {
try {
double scoreValue = Double.parseDouble(score);
if (scoreValue < 0 || scoreValue > 100) {
throw new NumberFormatException("成绩应在0-100之间");
}
// 将数据插入到数据库中
insertData(id, name, scoreValue);
// 提示插入成功
Alert alert = new Alert(AlertType.INFORMATION, "插入成功");
alert.showAndWait();
} catch (NumberFormatException e) {
// 成绩格式不正确
Alert alert = new Alert(AlertType.ERROR, "成绩格式不正确: " + e.getMessage());
alert.showAndWait();
}
}
}
private void insertData(String id, String name, double score) {
// TODO: 将数据插入到数据库中
}
public static void main(String[] args) {
launch(args);
}
}
```
在上面的代码中,我们使用了Alert对话框来提示用户输入的数据是否正确。如果数据不正确,会弹出错误提示框;如果数据正确,则会将数据插入到数据库中,并弹出成功提示框。
需要注意的是,在insertData方法中,我们需要使用JDBC来连接数据库,并将数据插入到student表中。以下是一个简单的示例,您可以根据自己的需求进行修改:
```java
private void insertData(String id, String name, double score) throws SQLException {
// 连接数据库
String url = "jdbc:mysql://localhost:3306/xsgl";
String user = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, user, password);
// 插入数据
String sql = "INSERT INTO student (id, name, score) VALUES (?, ?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setString(1, id);
stmt.setString(2, name);
stmt.setDouble(3, score);
stmt.executeUpdate();
// 关闭连接
stmt.close();
conn.close();
}
```
最后,您需要进行基本的测试,确保程序能够正常插入数据并提示用户。如果您有其他问题,欢迎继续向我提问。
相关推荐
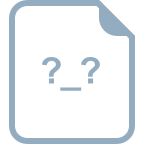
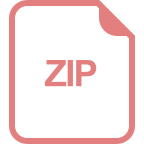
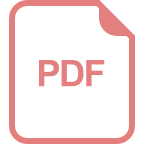










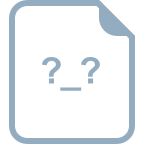
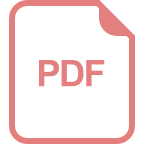
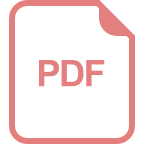
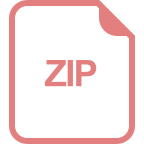