求助!java问题设有一个由10个英文单词构成的字符串数组,要求:(1)统计以字母w开头的单词数;(2)统计单词中含“or”字符串的单词数;(3)统计长度为3的单词数。
时间: 2024-11-06 09:13:26 浏览: 21
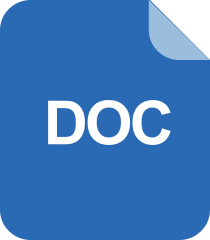
java常见问题(java 常见错误)

在Java中,你可以通过遍历字符串数组并使用内置的方法来解决这个问题。这里是一个简单的示例代码片段:
```java
import java.util.Arrays;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class WordCount {
public static void main(String[] args) {
String[] wordArray = {"walk", "world", "water", "work", "wave", "window", "warrior", "worm", "weather", "warm"};
// 统计以 'w' 开头的单词数
int wordsStartingWithW = countWordsStartingWith("w", wordArray);
System.out.println("单词以 'w' 开头的数量: " + wordsStartingWithW);
// 统计包含 'or' 的单词数
int wordsContainingOr = countWordsWithSubstring("or", wordArray);
System.out.println("包含 'or' 字符串的单词数量: " + wordsContainingOr);
// 统计长度为3的单词数
int threeLetterWords = countThreeLetterWords(wordArray);
System.out.println("长度为3的单词数量: " + threeLetterWords);
}
private static int countWordsStartingWith(String prefix, String[] words) {
int count = 0;
for (String word : words) {
if (word.startsWith(prefix)) {
count++;
}
}
return count;
}
private static int countWordsWithSubstring(String substring, String[] words) {
int count = 0;
Pattern pattern = Pattern.compile(substring, Pattern.CASE_INSENSITIVE);
for (String word : words) {
Matcher matcher = pattern.matcher(word);
if (matcher.find()) {
count++;
}
}
return count;
}
private static int countThreeLetterWords(String[] words) {
int count = 0;
for (String word : words) {
if (word.length() == 3) {
count++;
}
}
return count;
}
}
```
在这个代码中,`countWordsStartingWith`、`countWordsWithSubstring` 和 `countThreeLetterWords` 分别用于计算符合条件的单词数。运行这个程序,你会得到每个条件下的单词数量。
阅读全文
相关推荐
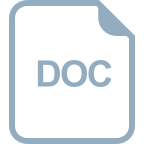
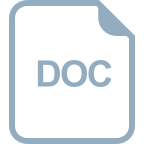
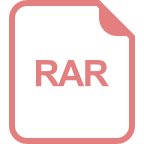
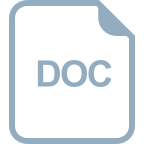
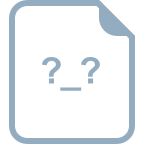
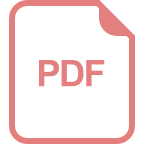
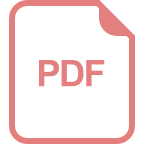
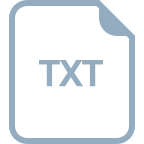
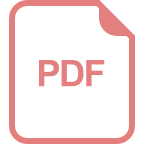
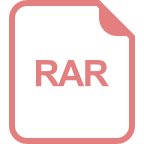
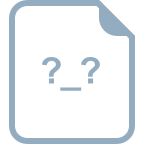
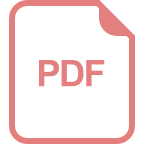
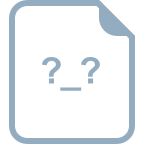
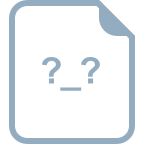