帮我用c++编写一个可运行的二叉树结构,实现“二叉树”的创建、输出二叉树先序遍历、中序遍历和后序遍历
时间: 2024-05-04 09:21:56 浏览: 106
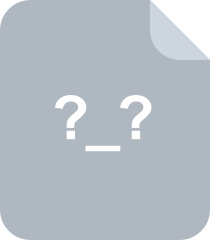
二叉树的先序、中序、后序、分层遍历C++实现

好的,这是一个简单的二叉树结构,包含了创建、先序遍历、中序遍历和后序遍历的实现。
```C++
#include <iostream>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
void preorder(TreeNode* root) {
if (root == NULL) return;
cout << root->val << " ";
preorder(root->left);
preorder(root->right);
}
void inorder(TreeNode* root) {
if (root == NULL) return;
inorder(root->left);
cout << root->val << " ";
inorder(root->right);
}
void postorder(TreeNode* root) {
if (root == NULL) return;
postorder(root->left);
postorder(root->right);
cout << root->val << " ";
}
TreeNode* create() {
int x;
cin >> x;
if (x == -1) return NULL;
TreeNode* root = new TreeNode(x);
root->left = create();
root->right = create();
return root;
}
int main() {
TreeNode* root = create();
cout << "preorder: ";
preorder(root);
cout << endl;
cout << "inorder: ";
inorder(root);
cout << endl;
cout << "postorder: ";
postorder(root);
cout << endl;
return 0;
}
```
这里的 `create()` 函数使用递归方式创建二叉树,输入 `-1` 表示当前节点为空。 `preorder()`、`inorder()` 和 `postorder()` 分别实现了二叉树的先序遍历、中序遍历和后序遍历。在 `main()` 函数中,我们先调用 `create()` 创建二叉树,然后输出三种遍历方式的结果。
阅读全文
相关推荐
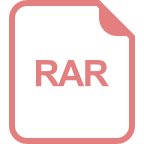
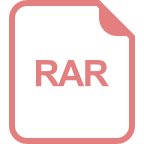










