java 正则匹配数组
时间: 2023-09-17 17:09:53 浏览: 217
可以使用Java的正则表达式来匹配数组。
例如,假设你有一个数组,其格式为:
```
int[] arr = {1, 2, 3, 4, 5};
```
你可以使用以下正则表达式来匹配它:
```
int\[\] arr = \{(\d+(, )?)+\};
```
该正则表达式的解释如下:
- `int\[\] arr =`:匹配字符串 "int[] arr = "
- `\{`:匹配左花括号 "{"
- `(\d+(, )?)+`:匹配一个或多个数字,后面可能跟一个逗号和一个空格的组合,重复多次
- `\}`:匹配右花括号 "}"
在Java中,你可以使用`Pattern`和`Matcher`类来实现正则表达式匹配。以下是一个示例代码:
```java
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String str = "int[] arr = {1, 2, 3, 4, 5};";
String regex = "int\\[\\] arr = \\{(\\d+(, )?)+\\}";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
System.out.println("Match found: " + matcher.group(0));
} else {
System.out.println("Match not found");
}
}
}
```
输出结果应该为:
```
Match found: int[] arr = {1, 2, 3, 4, 5}
```
注意,这只是一个简单的示例,实际应用中可能需要更复杂的正则表达式来匹配不同形式的数组。
阅读全文
相关推荐
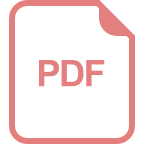
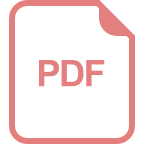
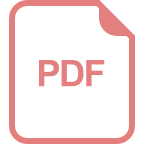















