用c#写一个五子棋程序
时间: 2023-12-03 08:04:58 浏览: 68
语言编程时,我们需要写出符合C语言语法规则的代码,通过编译器将代码转换成可执行文件进行运行。C语言是一门非常流行的编程语言,适用于各种应用场景,如系统编程、嵌入式开发等。C语言的特点是语法简洁,性能高效,可移植性好,易于学习和使用。在编程过程中,我们需要注意代码的正确性和效率,以及进行必要的调试和优化。
相关问题
用C#写一个五子棋程序设计
好的,下面是一个简单的五子棋程序的 C# 设计。这个程序只有基本功能,可以运行并且可以下棋。
首先,需要一个表示棋盘的二维数组,可以用 int 类型表示,0 表示空位,1 表示黑棋,2 表示白棋。定义如下:
```
int[,] board = new int[15, 15];
```
然后需要一个方法来显示棋盘,可以使用 Console.WriteLine() 方法,在控制台显示棋盘。定义如下:
```
void DrawBoard()
{
Console.WriteLine(" 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15");
for (int i = 0; i < 15; i++)
{
Console.Write((i + 1).ToString().PadLeft(2) + " ");
for (int j = 0; j < 15; j++)
{
switch (board[i, j])
{
case 0:
Console.Write("+ ");
break;
case 1:
Console.Write("● ");
break;
case 2:
Console.Write("○ ");
break;
}
}
Console.WriteLine();
}
}
```
然后需要一个方法来判断落子是否合法,即判断该位置是否为空。定义如下:
```
bool IsValidMove(int x, int y)
{
if (x < 0 || x >= 15 || y < 0 || y >= 15)
{
return false;
}
if (board[x, y] != 0)
{
return false;
}
return true;
}
```
接下来需要一个方法来判断游戏是否结束,即是否有五子连珠。这个方法比较复杂,需要判断横向、纵向、左上到右下、右上到左下四个方向是否有五子连珠。定义如下:
```
bool IsGameOver(int x, int y)
{
int color = board[x, y];
// 横向判断
int count = 0;
for (int i = x - 4; i <= x + 4; i++)
{
if (i < 0 || i >= 15)
{
continue;
}
if (board[i, y] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 纵向判断
count = 0;
for (int i = y - 4; i <= y + 4; i++)
{
if (i < 0 || i >= 15)
{
continue;
}
if (board[x, i] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 左上到右下判断
count = 0;
for (int i = -4; i <= 4; i++)
{
int tx = x + i;
int ty = y + i;
if (tx < 0 || tx >= 15 || ty < 0 || ty >= 15)
{
continue;
}
if (board[tx, ty] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 右上到左下判断
count = 0;
for (int i = -4; i <= 4; i++)
{
int tx = x + i;
int ty = y - i;
if (tx < 0 || tx >= 15 || ty < 0 || ty >= 15)
{
continue;
}
if (board[tx, ty] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
return false;
}
```
最后需要一个方法来进行游戏。该方法使用循环,交替下黑棋和白棋,每次落子后判断游戏是否结束。定义如下:
```
void PlayGame()
{
int player = 1;
while (true)
{
DrawBoard();
Console.WriteLine("轮到玩家 " + player + " 下棋,请输入坐标(如:3,4):");
string input = Console.ReadLine();
string[] inputs = input.Split(',');
int x = int.Parse(inputs[0]) - 1;
int y = int.Parse(inputs[1]) - 1;
if (!IsValidMove(x, y))
{
Console.WriteLine("无效落子,请重新输入!");
continue;
}
board[x, y] = player;
if (IsGameOver(x, y))
{
DrawBoard();
Console.WriteLine("玩家 " + player + " 获胜!");
break;
}
player = player == 1 ? 2 : 1;
}
}
```
最后在 Main 方法中调用 PlayGame() 方法即可开始游戏。完整代码如下:
```
using System;
class Program
{
static int[,] board = new int[15, 15];
static void Main(string[] args)
{
PlayGame();
}
static void DrawBoard()
{
Console.WriteLine(" 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15");
for (int i = 0; i < 15; i++)
{
Console.Write((i + 1).ToString().PadLeft(2) + " ");
for (int j = 0; j < 15; j++)
{
switch (board[i, j])
{
case 0:
Console.Write("+ ");
break;
case 1:
Console.Write("● ");
break;
case 2:
Console.Write("○ ");
break;
}
}
Console.WriteLine();
}
}
static bool IsValidMove(int x, int y)
{
if (x < 0 || x >= 15 || y < 0 || y >= 15)
{
return false;
}
if (board[x, y] != 0)
{
return false;
}
return true;
}
static bool IsGameOver(int x, int y)
{
int color = board[x, y];
// 横向判断
int count = 0;
for (int i = x - 4; i <= x + 4; i++)
{
if (i < 0 || i >= 15)
{
continue;
}
if (board[i, y] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 纵向判断
count = 0;
for (int i = y - 4; i <= y + 4; i++)
{
if (i < 0 || i >= 15)
{
continue;
}
if (board[x, i] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 左上到右下判断
count = 0;
for (int i = -4; i <= 4; i++)
{
int tx = x + i;
int ty = y + i;
if (tx < 0 || tx >= 15 || ty < 0 || ty >= 15)
{
continue;
}
if (board[tx, ty] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
// 右上到左下判断
count = 0;
for (int i = -4; i <= 4; i++)
{
int tx = x + i;
int ty = y - i;
if (tx < 0 || tx >= 15 || ty < 0 || ty >= 15)
{
continue;
}
if (board[tx, ty] == color)
{
count++;
}
else
{
count = 0;
}
if (count == 5)
{
return true;
}
}
return false;
}
static void PlayGame()
{
int player = 1;
while (true)
{
DrawBoard();
Console.WriteLine("轮到玩家 " + player + " 下棋,请输入坐标(如:3,4):");
string input = Console.ReadLine();
string[] inputs = input.Split(',');
int x = int.Parse(inputs[0]) - 1;
int y = int.Parse(inputs[1]) - 1;
if (!IsValidMove(x, y))
{
Console.WriteLine("无效落子,请重新输入!");
continue;
}
board[x, y] = player;
if (IsGameOver(x, y))
{
DrawBoard();
Console.WriteLine("玩家 " + player + " 获胜!");
break;
}
player = player == 1 ? 2 : 1;
}
}
}
```
用c#语言写个五子棋程序
语言编写程序时,需要注意以下几点:
1. C语言是一种高级语言,需要通过编译器将其转换成机器语言才能运行。
2. 在编写程序时,需要注意语法的正确性,例如括号的匹配、分号的使用等。
3. 变量的定义和使用需要注意数据类型的匹配,避免出现类型不匹配的错误。
4. 在编写程序时,需要注意程序的效率和可读性,避免出现死循环等问题。
5. 在调试程序时,可以使用调试工具来定位错误,例如使用断点和打印语句等。
总之,编写程序需要认真对待,遵循规范和良好的编程习惯,才能写出高质量的代码。
阅读全文
相关推荐
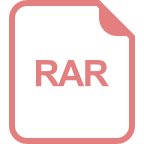
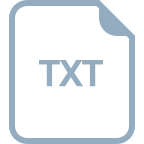
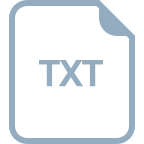


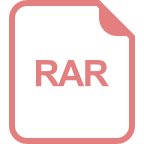
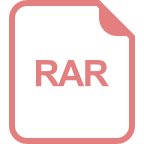
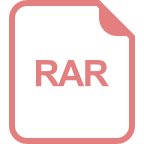
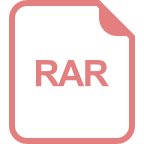


