半同步的消息机制中异步的send函数和同步的receive函数的实现代码
时间: 2024-02-23 11:00:36 浏览: 19
半同步的消息机制中,异步的 send 函数和同步的 receive 函数的实现代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#include <errno.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
// 异步发送消息函数
int asyn_send(int fd, char *buf, size_t size, int flags) {
ssize_t ret;
if ((ret = send(fd, buf, size, flags | MSG_DONTWAIT)) == -1 && errno == EAGAIN) {
// 如果 send 函数返回 EAGAIN 错误,则表示消息队列已满,可以不立即发送,等待下一次机会
return 0;
} else if (ret == -1) {
perror("send");
exit(1);
}
return 1;
}
// 同步接收消息函数
void sync_recv(int fd, struct msgbuf *buf, int type, int flags) {
ssize_t ret;
memset(buf, 0, sizeof(struct msgbuf));
// 循环接收消息,直到接收到指定类型的消息
while ((ret = msgrcv(fd, buf, MSG_SIZE, type, flags)) == -1 && errno == EINTR);
if (ret == -1) {
perror("msgrcv");
exit(1);
}
}
int main() {
int fd, flags;
struct msgbuf buf;
key_t key;
// 创建消息队列
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
if ((fd = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget");
exit(1);
}
// 异步发送消息
char *msg = "Hello, world!";
if (asyn_send(fd, msg, strlen(msg)+1, IPC_NOWAIT) == 0) {
printf("Message queue is full, try again later.\n");
}
// 同步接收消息
sync_recv(fd, &buf, 0, 0);
printf("Received message: %s\n", buf.mtext);
// 删除消息队列
if (msgctl(fd, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
该程序中,使用了异步的 send 函数和同步的 receive 函数来实现半同步的消息机制。在异步发送消息函数 asyn_send 中,使用了 send 函数的 MSG_DONTWAIT 标志来实现非阻塞发送,并通过返回值来判断消息队列是否已满。当消息队列已满时,函数会返回 0,表示暂时无法发送消息,需要等待下一次机会。
在同步接收消息函数 sync_recv 中,使用了 msgrcv 函数来进行阻塞式接收。当函数返回时,表示接收到了指定类型的消息,并将消息内容存储在 buf 中。
需要注意的是,由于异步发送消息函数和同步接收消息函数的实现方式不同,因此在使用时需要谨慎考虑,避免出现死锁或者消息丢失等问题。
相关推荐
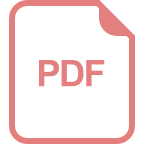














