用c获取获取文件名和后缀名
时间: 2024-05-15 19:19:16 浏览: 6
以下是用C语言获取文件名和后缀名的示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char filename[100];
printf("请输入文件名:");
scanf("%s", filename);
char *dot = strrchr(filename, '.');
if (dot) {
printf("文件名为:%.*s\n", (int)(dot - filename), filename);
printf("后缀名为:%s\n", dot + 1);
} else {
printf("该文件没有后缀名。\n");
}
return 0;
}
```
在上面的代码中,我们首先使用`scanf`函数获取用户输入的文件名,然后使用`strrchr`函数查找文件名中最后一个`.`字符,如果存在,就将`.`字符后面的部分作为后缀名输出,否则输出“该文件没有后缀名”。注意,我们使用了`strrchr`函数而不是`strchr`函数,是因为后缀名通常出现在文件名的末尾,而`strrchr`函数从字符串的末尾开始查找。另外,我们使用了`%.*s`格式化字符串输出文件名,这是因为我们只需要输出文件名中`.`字符之前的部分,而不是整个字符串。
相关问题
hutool获取文件后缀名
回答: 使用hutool获取文件后缀名的方法有多种。其中一种方法是使用FileNameUtil.getPrefix()方法。例如,如果文件路径为"c:\\xiaoxuzhu\\hutool1.jpg",可以使用以下代码获取文件后缀名:
String filePath = "c:\\xiaoxuzhu\\hutool1.jpg";
File file = FileUtil.file(filePath);
String suffix = FileNameUtil.getPrefix(file);
System.out.println(suffix);
这将输出"jpg"作为文件的后缀名。\[1\]
另一种方法是使用FileNameUtil.mainName()方法。例如,可以使用以下代码获取文件后缀名:
String filePath = "c:\\xiaoxuzhu\\hutool1.jpg";
String suffix = FileNameUtil.mainName(filePath);
System.out.println(suffix);
这也将输出"jpg"作为文件的后缀名。\[3\]
请注意,以上代码示例中的文件路径和文件名仅供参考,您需要根据实际情况修改文件路径和文件名。\[2\]
#### 引用[.reference_title]
- *1* *2* *3* [13《hutool实战》:FileNameUtil 文件名工具(JAVA 小虚竹)](https://blog.csdn.net/shi_hong_fei_hei/article/details/122675130)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
用Csharp写获取文件夹中文件并根据后缀名获取文件
以下是一个示例代码,使用C#语言获取文件夹中的文件并根据后缀名获取文件:
```
using System;
using System.IO;
namespace GetFilesByExtension
{
class Program
{
static void Main(string[] args)
{
string folderPath = @"C:\Users\username\Desktop\testFolder"; //文件夹路径
string extension = ".txt"; //文件后缀名
try
{
//获取指定后缀名的所有文件
string[] files = Directory.GetFiles(folderPath, "*" + extension);
//遍历所有文件并输出文件名
foreach (string file in files)
{
Console.WriteLine(Path.GetFileName(file));
}
}
catch (IOException ex)
{
Console.WriteLine("Error: " + ex.Message);
}
Console.ReadLine();
}
}
}
```
在此示例代码中,我们使用 `Directory.GetFiles()` 方法来获取指定文件夹中所有具有指定后缀名的文件。然后,我们使用 `foreach` 循环遍历所有文件并输出它们的文件名。
请注意,`Directory.GetFiles()` 方法的第一个参数是文件夹路径,第二个参数是文件名搜索模式。在此示例中,我们将搜索模式设置为 `*` + `extension`,以便找到所有具有指定后缀名的文件。
相关推荐
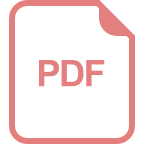
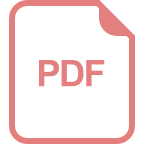
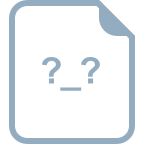












