将df输出的第一行的进行抽取,写入file2中
时间: 2024-02-23 15:58:39 浏览: 14
可以使用以下命令将df输出的第一行写入file2中:
```
df | head -n 1 > file2
```
这个命令中,`df`命令用于显示磁盘空间使用情况,`head -n 1`命令用于只显示第一行,`>`符号用于将输出结果重定向到`file2`文件中。这样,`file2`文件中就只包含了`df`输出结果的第一行。
相关问题
python将df分组后批量写入excel中写入倒数第2个新SHEET
好的,让我来回答您的问题。首先,您需要使用Python的pandas库进行数据分组,然后使用xlwt或openpyxl库将数据批量写入Excel中的倒数第二个sheet。以下是Python代码示例:
```python
import pandas as pd
import xlwt # 用于写入xls文件
from openpyxl import Workbook # 用于写入xlsx文件
# 读取数据
df = pd.read_excel('data.xlsx')
# 按某一列分组
grouped = df.groupby('col')
# 将每个分组写入Excel中的新sheet
book = xlwt.Workbook()
for name, group in grouped:
sheet = book.add_sheet(name) # 以分组名称创建新sheet
for i in range(len(group.columns)):
sheet.write(0, i, group.columns[i]) # 将列名写入第一行
for j in range(len(group)):
sheet.write(j+1, i, group.iloc[j,i]) # 将数据写入每一行中
# 将新sheet插入到倒数第二个位置
book.add_sheet('new_sheet', -1)
# 将Excel保存到文件
book.save('result.xls')
```
如果您需要将数据写入“xlsx”格式的Excel文件,则可以使用openpyxl库。以下是使用该库的示例代码:
```python
import pandas as pd
from openpyxl import Workbook
# 读取数据
df = pd.read_excel('data.xlsx')
# 按某一列分组
grouped = df.groupby('col')
# 将每个分组写入Excel中的新sheet
book = Workbook()
for name, group in grouped:
sheet = book.create_sheet(name) # 以分组名称创建新sheet
for i in range(len(group.columns)):
sheet.cell(row=1, column=i+1, value=str(group.columns[i])) # 将列名写入第一行
for j in range(len(group)):
sheet.cell(row=j+2, column=i+1, value=str(group.iloc[j,i])) # 将数据写入每一行中
# 将新sheet插入到倒数第二个位置
sheet = book.create_sheet('new_sheet', -2)
# 将Excel保存到文件
book.save('result.xlsx')
```
注意,这些示例代码仅供参考,您可能需要根据您的具体数据格式和要求进行修改。
df.to_excel写入第一行
您可以使用 `pandas` 库的 `ExcelWriter` 和 `to_excel` 方法来实现写入第一行。具体操作如下:
```python
import pandas as pd
# 创建一个数据框 df
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# 创建一个 ExcelWriter 对象
writer = pd.ExcelWriter('output.xlsx')
# 将数据框 df 写入 Excel 文件中,从第二行开始写入
df.to_excel(writer, startrow=1, index=False)
# 获取 Excel 文件中的工作表对象
worksheet = writer.sheets['Sheet1']
# 在第一行插入数据
worksheet.write(0, 0, 'Hello')
worksheet.write(0, 1, 'World')
# 保存并关闭 ExcelWriter 对象
writer.save()
```
在上述代码中,`startrow=1` 表示从第二行开始写入数据,因此我们可以在第一行插入数据。`index=False` 表示不将数据框中的行索引写入到 Excel 文件中。`worksheet.write(0, 0, 'Hello')` 和 `worksheet.write(0, 1, 'World')` 分别在第一行第一列和第二列插入字符串 `'Hello'` 和 `'World'`。
相关推荐
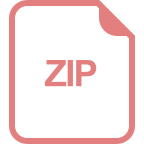
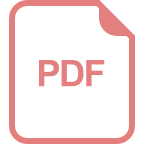












