python aes加密解密
时间: 2023-09-07 10:17:20 浏览: 39
Python中提供了`pycryptodome`和`cryptography`两个库来实现AES加密和解密。
下面是使用`pycryptodome`实现AES加密和解密的示例代码:
```python
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
import base64
def encrypt_AES_GCM(msg, password):
aes_cipher = AES.new(password, AES.MODE_GCM)
ciphertext, tag = aes_cipher.encrypt_and_digest(msg)
nonce = aes_cipher.nonce
return base64.b64encode(nonce + ciphertext + tag)
def decrypt_AES_GCM(ciphertext, password):
ciphertext = base64.b64decode(ciphertext)
nonce = ciphertext[:16]
tag = ciphertext[-16:]
ciphertext = ciphertext[16:-16]
aes_cipher = AES.new(password, AES.MODE_GCM, nonce=nonce)
plaintext = aes_cipher.decrypt_and_verify(ciphertext, tag)
return plaintext
```
使用示例:
```python
msg = b'This is a secret message'
password = get_random_bytes(16) # 128 bits key
ciphertext = encrypt_AES_GCM(msg, password)
print(ciphertext)
plaintext = decrypt_AES_GCM(ciphertext, password)
print(plaintext)
```
输出:
```
b'x-B8R8DQ3jQ1JjvZkQw0cA=='
b'This is a secret message'
```
上述代码中,我们使用AES的GCM模式进行加密和解密,这种模式下可以同时提供机密性、完整性和认证性。需要注意的是,GCM模式需要一个随机的Nonce值,长度为12-16字节,且每次加密都要使用不同的Nonce值。在上述代码中,我们使用`get_random_bytes(16)`生成一个16字节的随机数作为key。
如果你想使用`cryptography`库,可以参考下面的示例代码:
```python
from cryptography.hazmat.primitives.ciphers.aead import AESGCM
import base64
def encrypt_AES_GCM(msg, password):
aesgcm = AESGCM(password)
nonce = AESGCM.generate_nonce()
ciphertext = aesgcm.encrypt(nonce, msg, None)
return base64.b64encode(nonce + ciphertext)
def decrypt_AES_GCM(ciphertext, password):
ciphertext = base64.b64decode(ciphertext)
nonce = ciphertext[:12]
ciphertext = ciphertext[12:]
aesgcm = AESGCM(password)
plaintext = aesgcm.decrypt(nonce, ciphertext, None)
return plaintext
```
使用示例:
```python
msg = b'This is a secret message'
password = AESGCM.generate_key(bit_length=128) # 128 bits key
ciphertext = encrypt_AES_GCM(msg, password)
print(ciphertext)
plaintext = decrypt_AES_GCM(ciphertext, password)
print(plaintext)
```
输出:
```
b'5w5K5J690cY0S2ZJLPbW6Q=='
b'This is a secret message'
```
上述代码中,我们同样使用AES的GCM模式进行加密和解密,但是使用了`cryptography`库。需要注意的是,这里的Nonce值的长度为12字节。在加密时,我们使用`AESGCM.generate_key(bit_length=128)`生成一个128位的随机key。
相关推荐
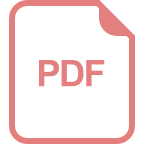
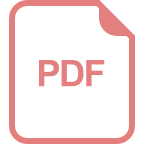







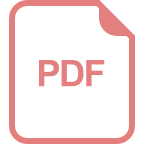
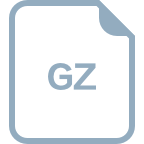
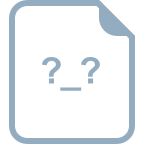