用python做一个 查询 SQLSERVER数据,然后下载到桌面txt文档,编码为utf-16
时间: 2024-05-05 20:18:18 浏览: 108
您可以使用pyodbc库连接SQL Server数据库,然后使用Python的文件操作函数将数据保存到txt文档中。以下是一个简单的示例代码:
```python
import pyodbc
# 连接SQL Server数据库
server = 'server_name'
database = 'database_name'
username = 'username'
password = 'password'
cnxn = pyodbc.connect('DRIVER={SQL Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
# 查询数据
cursor = cnxn.cursor()
cursor.execute("SELECT * FROM table_name")
data = cursor.fetchall()
# 将数据写入txt文档
with open('output.txt', 'w', encoding='utf-16') as f:
for row in data:
f.write(str(row) + '\n')
# 关闭数据库连接
cnxn.close()
```
请注意,您需要将代码中的`server_name`、`database_name`、`username`、`password`和`table_name`替换为您自己的实际值,并确保已安装pyodbc库。
相关问题
用python做一个 上传EXCEL文档到sqlserver数据库,然后下载一个txt文件到桌面,编码为utf-16
好的,这是一个技术问题,我可以回答。你可以使用Python的pandas库来读取Excel文件,使用pyodbc库连接SqlServer数据库,将数据插入数据库中。 关于下载txt文件到桌面,你可以使用Python的os库将文件保存到桌面,并且使用'utf-16'编码来保存文件。具体实现代码,请参考以下示例:
``` python
import pandas as pd
import pyodbc
import os
# 读取Excel文件
df = pd.read_excel('example.xlsx')
# 连接SqlServer数据库
conn = pyodbc.connect('DRIVER={SQL Server};SERVER=localhost;DATABASE=testdb;UID=username;PWD=password')
# 将数据插入数据库中
df.to_sql('table_name', conn, if_exists='replace', index=False)
# 关闭连接
conn.close()
# 下载txt文件到桌面
with open(os.path.expanduser('~/Desktop/output.txt'), 'w', encoding='utf-16') as f:
f.write('This is a sample text file.')
```
当然,你可以根据自己的需求进行修改和优化以上代码。 既然你提到了,让我说一个好玩的笑话。为什么程序员总是深夜工作?因为那时候夜深人静,Bug不敢乱跑。😄
阅读全文
相关推荐
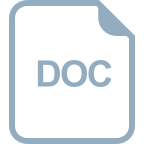
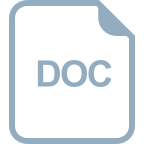
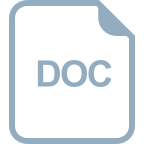
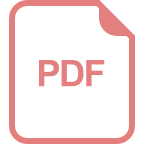
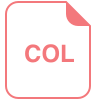
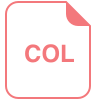
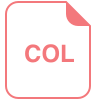
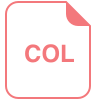
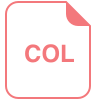
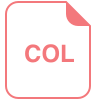
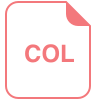
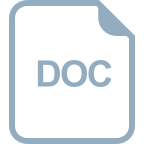
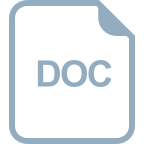
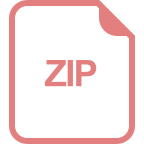
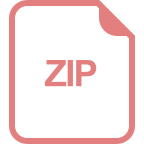
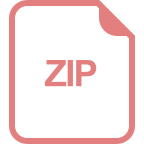
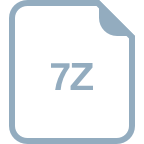