html中使用Thymeleaf绘制销售单表格,每个表格存14行数据,超过14行新的相同表格,同时计算销售单表格中的金额乘以单价并转换成文字大写
时间: 2024-05-02 10:17:31 浏览: 78
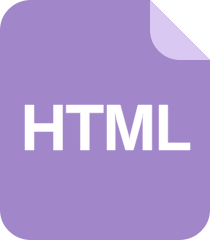
html表格设计

首先,我们可以使用Thymeleaf中的循环来绘制表格。假设我们有一个名为sales的List对象,其中每个元素都包含14行数据,我们可以这样绘制表格:
```html
<table>
<thead>
<tr>
<th>商品名称</th>
<th>数量</th>
<th>单价</th>
<th>金额</th>
</tr>
</thead>
<tbody>
<tr th:each="item, itemIndex : ${sales}">
<td th:text="${item.name}"></td>
<td th:text="${item.quantity}"></td>
<td th:text="${item.unitPrice}"></td>
<td th:text="${item.quantity * item.unitPrice}"></td>
</tr>
</tbody>
</table>
```
对于超过14行的数据,我们可以使用Thymeleaf中的条件判断来绘制多个表格:
```html
<div th:each="salesChunk : ${#lists.chunk(sales, 14)}">
<table>
<thead>
<tr>
<th>商品名称</th>
<th>数量</th>
<th>单价</th>
<th>金额</th>
</tr>
</thead>
<tbody>
<tr th:each="item, itemIndex : ${salesChunk}">
<td th:text="${item.name}"></td>
<td th:text="${item.quantity}"></td>
<td th:text="${item.unitPrice}"></td>
<td th:text="${item.quantity * item.unitPrice}"></td>
</tr>
</tbody>
</table>
</div>
```
最后,对于金额乘以单价并转换成文字大写的需求,我们可以使用Java中的工具类来实现。比如,我们可以使用Apache Commons Lang库中的NumberToWords类:
```java
import org.apache.commons.lang3.text.WordUtils;
import java.math.BigDecimal;
public class NumberToWordsConverter {
public static String toWords(BigDecimal number) {
String words = NumberToWords.convert(number.longValue());
return WordUtils.capitalize(words) + "元整";
}
}
```
然后,在Thymeleaf模板中使用该工具类进行金额的转换:
```html
<td th:text="${#numbers.formatDecimal(item.quantity * item.unitPrice, 2, 'COMMA_POINT')}"></td>
<td th:text="${T(com.example.NumberToWordsConverter).toWords(item.quantity * item.unitPrice)}"></td>
```
其中,`#numbers.formatDecimal`用于格式化金额为小数点分隔符格式,`T()`用于调用工具类中的静态方法。
阅读全文
相关推荐
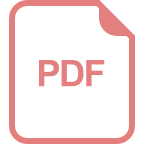


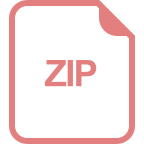
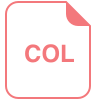
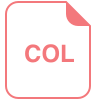
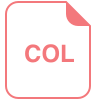

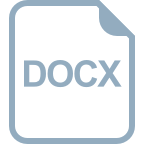
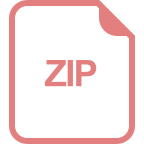
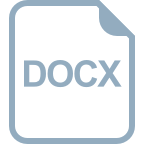
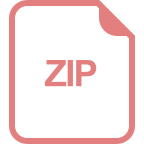
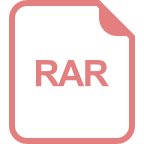
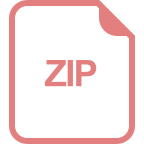