java有什么办法可以把一串很长的字符串变短,然后还能完全还原回来
时间: 2024-01-27 12:03:26 浏览: 148
Java中可以使用压缩算法对字符串进行压缩,从而将长字符串变短,例如使用gzip或者deflate算法。对于压缩后的字符串,可以使用相应的解压算法来还原原始字符串。在Java中,可以使用java.util.zip包中的类来进行压缩和解压操作,例如:
``` java
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.zip.DataFormatException;
import java.util.zip.Deflater;
import java.util.zip.Inflater;
public class StringCompressor {
public static byte[] compress(String str) throws IOException {
byte[] input = str.getBytes("UTF-8");
Deflater deflater = new Deflater();
deflater.setInput(input);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream(input.length);
deflater.finish();
byte[] buffer = new byte[1024];
while (!deflater.finished()) {
int count = deflater.deflate(buffer);
outputStream.write(buffer, 0, count);
}
outputStream.close();
byte[] output = outputStream.toByteArray();
return output;
}
public static String decompress(byte[] compressed) throws IOException, DataFormatException {
Inflater inflater = new Inflater();
inflater.setInput(compressed);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream(compressed.length);
byte[] buffer = new byte[1024];
while (!inflater.finished()) {
int count = inflater.inflate(buffer);
outputStream.write(buffer, 0, count);
}
outputStream.close();
byte[] output = outputStream.toByteArray();
return new String(output, "UTF-8");
}
}
```
使用示例:
``` java
String original = "这是一段很长的字符串,我们将对其进行压缩和解压缩操作";
byte[] compressed = StringCompressor.compress(original);
String decompressed = StringCompressor.decompress(compressed);
System.out.println(decompressed.equals(original)); // 输出 true
```
上述代码演示了如何使用gzip算法对字符串进行压缩和解压缩。注意,在实际应用中,压缩和解压缩的过程中可能会出现异常,需要进行异常处理。
阅读全文
相关推荐


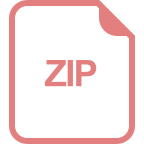
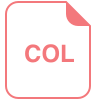
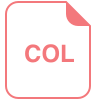
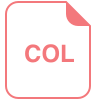
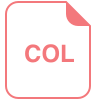
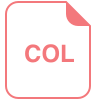
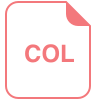
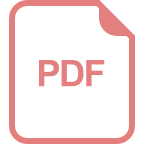
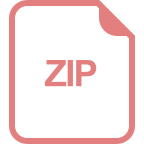
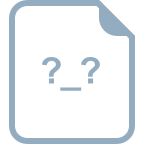
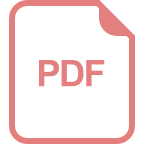
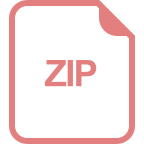
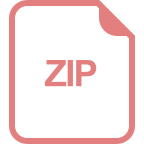