基于javaweb写一个学生管理系统
时间: 2023-09-21 11:02:46 浏览: 53
好的,下面是一个简单的JavaWeb学生管理系统的实现过程:
1. 确定系统需求和功能模块,例如学生信息管理、课程管理、成绩管理等。
2. 创建数据库,建立学生表、课程表和成绩表,并建立表之间的关系。
3. 使用JSP和Servlet技术建立前端页面和后端控制器,实现用户输入数据、查询数据、修改数据和删除数据等操作。
4. 使用JDBC技术连接数据库,实现数据的增删改查操作。
5. 使用MVC模式进行代码的分层,将业务逻辑、数据访问、视图显示分开,使系统具有良好的可维护性。
下面是一个简单的示例代码:
1. 学生实体类:
```
public class Student {
private int id; // 学生ID
private String name; // 学生姓名
private int age; // 学生年龄
// 构造方法
public Student(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
// getter和setter方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
```
2. 数据库连接工具类:
```
public class DBUtil {
private static final String URL = "jdbc:mysql://localhost:3306/student"; // 数据库连接地址
private static final String USERNAME = "root"; // 数据库用户名
private static final String PASSWORD = "123456"; // 数据库密码
// 获取数据库连接
public static Connection getConnection() throws SQLException, ClassNotFoundException {
Class.forName("com.mysql.jdbc.Driver"); // 加载数据库驱动
return DriverManager.getConnection(URL, USERNAME, PASSWORD); // 获取数据库连接
}
// 关闭数据库连接
public static void closeConnection(Connection conn, Statement stmt, ResultSet rs) throws SQLException {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
}
}
```
3. 学生DAO类:
```
public class StudentDAO {
// 添加学生信息
public void add(Student student) throws SQLException, ClassNotFoundException {
Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement("insert into student(id, name, age) values (?, ?, ?)");
pstmt.setInt(1, student.getId());
pstmt.setString(2, student.getName());
pstmt.setInt(3, student.getAge());
pstmt.executeUpdate();
DBUtil.closeConnection(conn, pstmt, null);
}
// 删除学生信息
public void delete(int id) throws SQLException, ClassNotFoundException {
Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement("delete from student where id = ?");
pstmt.setInt(1, id);
pstmt.executeUpdate();
DBUtil.closeConnection(conn, pstmt, null);
}
// 修改学生信息
public void update(Student student) throws SQLException, ClassNotFoundException {
Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement("update student set name = ?, age = ? where id = ?");
pstmt.setString(1, student.getName());
pstmt.setInt(2, student.getAge());
pstmt.setInt(3, student.getId());
pstmt.executeUpdate();
DBUtil.closeConnection(conn, pstmt, null);
}
// 查询所有学生信息
public List<Student> findAll() throws SQLException, ClassNotFoundException {
List<Student> students = new ArrayList<>();
Connection conn = DBUtil.getConnection();
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("select * from student");
while (rs.next()) {
Student student = new Student(rs.getInt("id"), rs.getString("name"), rs.getInt("age"));
students.add(student);
}
DBUtil.closeConnection(conn, stmt, rs);
return students;
}
// 根据ID查询学生信息
public Student findById(int id) throws SQLException, ClassNotFoundException {
Student student = null;
Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement("select * from student where id = ?");
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
student = new Student(rs.getInt("id"), rs.getString("name"), rs.getInt("age"));
}
DBUtil.closeConnection(conn, pstmt, rs);
return student;
}
}
```
4. 控制器Servlet类:
```
@WebServlet("/student")
public class StudentServlet extends HttpServlet {
private StudentDAO studentDAO;
public void init() {
studentDAO = new StudentDAO();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if (action == null) {
action = "list";
}
switch (action) {
case "list":
list(request, response);
break;
case "add":
add(request, response);
break;
case "delete":
delete(request, response);
break;
case "edit":
edit(request, response);
break;
case "update":
update(request, response);
break;
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
// 列出所有学生信息
private void list(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
List<Student> students = studentDAO.findAll();
request.setAttribute("students", students);
request.getRequestDispatcher("/list.jsp").forward(request, response);
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
// 添加学生信息
private void add(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
int age = Integer.parseInt(request.getParameter("age"));
Student student = new Student(id, name, age);
try {
studentDAO.add(student);
response.sendRedirect("student?action=list");
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
// 删除学生信息
private void delete(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try {
studentDAO.delete(id);
response.sendRedirect("student?action=list");
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
// 修改学生信息
private void edit(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try {
Student student = studentDAO.findById(id);
request.setAttribute("student", student);
request.getRequestDispatcher("/edit.jsp").forward(request, response);
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
// 更新学生信息
private void update(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
int age = Integer.parseInt(request.getParameter("age"));
Student student = new Student(id, name, age);
try {
studentDAO.update(student);
response.sendRedirect("student?action=list");
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
5. 前端页面:
list.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统</title>
</head>
<body>
<h1>学生列表</h1>
<a href="add.jsp">添加学生</a>
<table border="1">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
<c:forEach var="student" items="${students}">
<tr>
<td><c:out value="${student.id}"/></td>
<td><c:out value="${student.name}"/></td>
<td><c:out value="${student.age}"/></td>
<td>
<a href="student?action=edit&id=${student.id}">修改</a>
<a href="student?action=delete&id=${student.id}">删除</a>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
add.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加学生信息</title>
</head>
<body>
<h1>添加学生信息</h1>
<form action="student?action=add" method="post">
<label>ID:</label>
<input type="text" name="id"><br>
<label>姓名:</label>
<input type="text" name="name"><br>
<label>年龄:</label>
<input type="text" name="age"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
edit.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>修改学生信息</title>
</head>
<body>
<h1>修改学生信息</h1>
<form action="student?action=update" method="post">
<label>ID:</label>
<input type="text" name="id" value="${student.id}" readonly><br>
<label>姓名:</label>
<input type="text" name="name" value="${student.name}"><br>
<label>年龄:</label>
<input type="text" name="age" value="${student.age}"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
以上就是一个简单的JavaWeb学生管理系统的实现过程。当然,这只是一个基础的示例,实际开发中还需要考虑数据校验、权限控制、分页显示等功能。
相关推荐
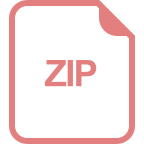
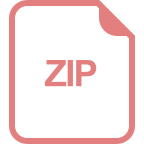
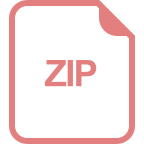














