.编写对任意输入的三个数进行由大到小输出的函数模板,并使用该模板
时间: 2023-09-10 17:04:49 浏览: 43
### 回答1:
以下是对任意输入的三个数进行由大到小输出的函数模板的实现:
```c++
#include <iostream>
using namespace std;
template <typename T>
void sortThree(T a, T b, T c) {
if (a > b) {
swap(a, b);
}
if (b > c) {
swap(b, c);
}
if (a > b) {
swap(a, b);
}
cout << c << " " << b << " " << a << endl;
}
int main() {
sortThree(3, 2, 1); // 3 2 1
sortThree(1.1, 2.2, 3.3); // 3.3 2.2 1.1
sortThree('c', 'b', 'a'); // c b a
return 0;
}
```
这里使用了模板函数,可以处理不同类型的输入参数,例如整数、浮点数和字符。调用 `sortThree()` 函数时,输入三个参数,函数会根据大小比较排序后输出。
### 回答2:
编写一个函数模板,名为sortDescend,接收三个参数并将它们由大到小输出。
```cpp
#include <iostream>
template<typename T>
void sortDescend(T a, T b, T c) {
T arr[] = {a, b, c};
int size = sizeof(arr) / sizeof(T);
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j] < arr[j + 1]) {
T temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
}
int main() {
int a, b, c;
std::cout << "请输入三个整数: ";
std::cin >> a >> b >> c;
sortDescend(a, b, c);
return 0;
}
```
在main函数中,我们首先获取输入的三个整数a,b,c,然后调用sortDescend函数模板,并将这三个整数作为参数传递给它。
运行程序后,程序会按照从大到小的顺序输出这三个整数。
### 回答3:
可以使用C++编写一个函数模板实现对任意输入的三个数进行由大到小输出的功能。
```c++
#include <iostream>
using namespace std;
template<typename T>
void sort_descending(T a, T b, T c) {
T arr[3] = { a, b, c };
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2 - i; j++) {
if (arr[j] < arr[j + 1]) {
T temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
cout << "由大到小排序结果为:" << endl;
for (int i = 0; i < 3; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
int main() {
int a, b, c;
cout << "请输入三个整数:" << endl;
cin >> a >> b >> c;
sort_descending(a, b, c);
double d, e, f;
cout << "请输入三个浮点数:" << endl;
cin >> d >> e >> f;
sort_descending(d, e, f);
return 0;
}
```
以上的代码是一个完整的程序。其中,template<typename T>定义了一个函数模板,该模板可以接受任意类型的参数。sort_descending函数用来对传入的三个参数进行由大到小的排序,并输出结果。
在main函数中,分别输入三个整数和三个浮点数,并调用sort_descending函数进行排序输出。
希望对你有帮助!
相关推荐
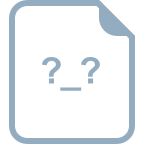
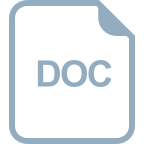














