用ajax访问请求数据,返回数据添加到bootstrap表格中,每一行添加按钮
时间: 2023-05-28 19:03:51 浏览: 224
可以按照以下步骤进行操作:
1. 使用jQuery的ajax方法向后端发送数据请求,并在成功回调函数中处理返回的数据。例如:
```javascript
$.ajax({
url: 'your_api_url',
type: 'GET',
dataType: 'json',
success: function(data) {
// 处理返回的数据
},
error: function(xhr, status, error) {
console.log('Error: ' + error.message);
}
});
```
2. 在成功回调函数中,使用jQuery的each方法遍历返回的数据,生成表格的每一行,并添加按钮。例如:
```javascript
$.each(data, function(index, item) {
var tr = $('<tr>');
tr.append('<td>' + item.name + '</td>');
tr.append('<td>' + item.age + '</td>');
tr.append('<td><button type="button" class="btn btn-primary">按钮</button></td>');
$('#table-body').append(tr);
});
```
3. 在HTML中添加一个空的表格,并给表格添加表头和表体。例如:
```html
<table class="table">
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody id="table-body"></tbody>
</table>
```
4. 最后,将以上代码整合到一个函数中,然后在页面加载完成后调用该函数即可。例如:
```javascript
$(function() {
function loadData() {
$.ajax({
url: 'your_api_url',
type: 'GET',
dataType: 'json',
success: function(data) {
$('#table-body').empty();
$.each(data, function(index, item) {
var tr = $('<tr>');
tr.append('<td>' + item.name + '</td>');
tr.append('<td>' + item.age + '</td>');
tr.append('<td><button type="button" class="btn btn-primary">按钮</button></td>');
$('#table-body').append(tr);
});
},
error: function(xhr, status, error) {
console.log('Error: ' + error.message);
}
});
}
loadData();
});
```
阅读全文
相关推荐
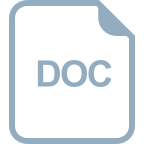
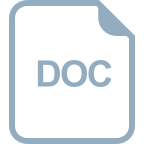
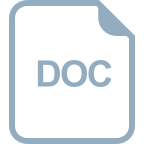


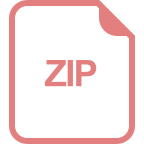
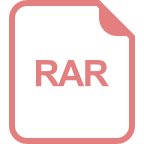
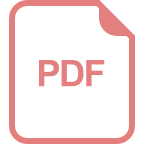
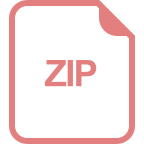
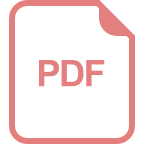
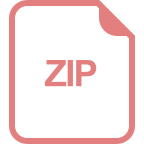
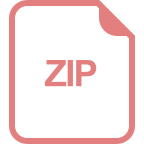
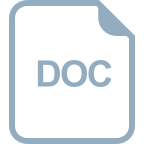
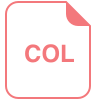
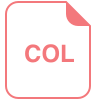



