建立一个链表,每个节点包括:学号、姓名、性别、年龄。输入一个年龄,如果链表中有与此年龄相同的节点,则将此节点删去
时间: 2024-04-30 20:22:48 浏览: 120
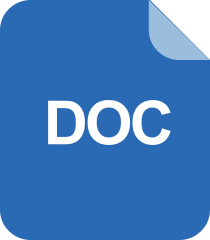
C语言课程设计
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct student {
int id;
char name[20];
char gender[5];
int age;
struct student* next;
} Student;
Student* create_student(int id, char* name, char* gender, int age) {
Student* new_student = (Student*)malloc(sizeof(Student));
new_student->id = id;
strcpy(new_student->name, name);
strcpy(new_student->gender, gender);
new_student->age = age;
new_student->next = NULL;
return new_student;
}
Student* add_student(Student* head, int id, char* name, char* gender, int age) {
Student* new_student = create_student(id, name, gender, age);
if (head == NULL) {
head = new_student;
} else {
Student* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_student;
}
return head;
}
Student* delete_student(Student* head, int age) {
if (head == NULL) {
return NULL;
}
Student* current = head;
Student* previous = NULL;
while (current != NULL) {
if (current->age == age) {
if (previous == NULL) {
head = current->next;
} else {
previous->next = current->next;
}
free(current);
current = previous == NULL ? head : previous->next;
} else {
previous = current;
current = current->next;
}
}
return head;
}
void print_student(Student* student) {
printf("ID: %d, Name: %s, Gender: %s, Age: %d\n", student->id, student->name, student->gender, student->age);
}
void print_all_students(Student* head) {
if (head == NULL) {
printf("The list is empty.\n");
} else {
Student* current = head;
while (current != NULL) {
print_student(current);
current = current->next;
}
}
}
void free_all_students(Student* head) {
Student* current = head;
while (current != NULL) {
Student* next = current->next;
free(current);
current = next;
}
}
int main() {
int id, age;
char name[20], gender[5];
Student* head = NULL;
printf("Please enter student information (ID, Name, Gender, Age), enter -1 to stop.\n");
while (1) {
printf("ID: ");
scanf("%d", &id);
if (id == -1) {
break;
}
printf("Name: ");
scanf("%s", name);
printf("Gender: ");
scanf("%s", gender);
printf("Age: ");
scanf("%d", &age);
head = add_student(head, id, name, gender, age);
}
printf("Please enter an age to delete: ");
scanf("%d", &age);
head = delete_student(head, age);
printf("The remaining students are:\n");
print_all_students(head);
free_all_students(head);
return 0;
}
```
阅读全文
相关推荐
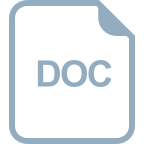
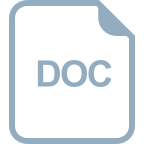











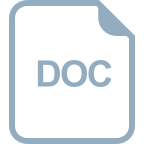
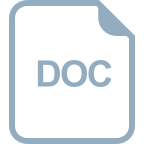
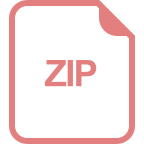