请用python语言编写一段请求网页的程序
时间: 2024-06-10 22:07:31 浏览: 95
好的,我可以为您提供代码示例:
```python
import requests
url = 'https://www.example.com'
response = requests.get(url)
html = response.text
print(html)
```
这段代码使用了 Python 的 requests 库来发送 GET 请求,并将响应的 HTML 文本打印输出。当然,您需要将 `url` 变量替换为您想要请求的实际网址。
相关问题
请使用python语言编写一段事例代码,验证Apache Thrift发送HTTP请求,使用Apache Spark接收和处理响应?
下面是一个简单的示例代码,演示如何使用Apache Thrift和Apache Spark:
1. 首先安装必要的库:Apache Thrift和PySpark
```
pip install thrift
pip install pyspark
```
2. 创建一个Thrift服务,用于处理HTTP请求,并将响应发送给Spark:
```python
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
from thrift.server import TServer
from http_handler import HttpHandler
# 创建一个Thrift处理程序
handler = HttpHandler()
# 创建一个Thrift服务
processor = HttpProcessor.Processor(handler)
# 监听端口并启动服务
transport = TSocket.TServerSocket(port=9090)
tfactory = TTransport.TBufferedTransportFactory()
pfactory = TBinaryProtocol.TBinaryProtocolFactory()
server = TServer.TThreadedServer(processor, transport, tfactory, pfactory)
print('Starting the server...')
server.serve()
```
3. 创建一个Spark应用程序,用于接收和处理Thrift服务的响应:
```python
from pyspark.sql import SparkSession
# 创建一个SparkSession
spark = SparkSession.builder.appName('ThriftResponse').getOrCreate()
# 读取Thrift服务响应
df = spark.read.format('thrift').load('http://localhost:9090')
# 处理响应数据
df.show()
# 关闭SparkSession
spark.stop()
```
4. 定义一个Thrift处理程序,用于处理HTTP请求并发送响应:
```python
from http_server import HttpServer
class HttpHandler(HttpServer.Iface):
def handle_request(self, url):
# 处理HTTP请求
response = requests.get(url)
# 将响应发送给Spark
transport = TSocket.TSocket('localhost', 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = HttpProcessor.Client(protocol)
transport.open()
client.send_response(response.content)
transport.close()
```
5. 最后,运行Thrift服务和Spark应用程序:
```
python thrift_server.py &
python spark_app.py
```
这个简单的示例演示了如何使用Apache Thrift和Apache Spark处理HTTP请求和响应。当Thrift服务接收到HTTP请求时,它将处理请求并向Spark发送响应。然后,Spark应用程序将读取响应数据并进行处理。
用Python语言编写一段爬虫代码
好的,以下是一个简单的爬虫代码,用于爬取指定网站的标题和链接:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
print(link.string)
```
这个爬虫通过requests库发送HTTP请求获取网页内容,然后使用BeautifulSoup库解析网页内容,从中提取出所有的链接和标题,并打印出来。当然,具体的爬取方式和目标网站的结构有关,需要根据实际情况做出相应的调整。
阅读全文
相关推荐
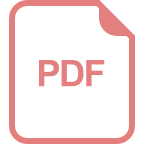




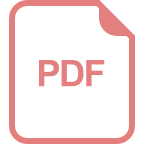
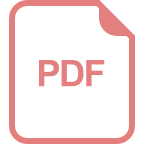
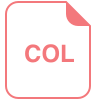






