java使用minio分片上传,获取到分片上传预签名地址后,如何调用后台接口上传分片(不能通过前台直接上传),java代码+vue代码实现
时间: 2024-01-29 09:08:08 浏览: 176
在Java中使用Minio进行分片上传,可以通过以下步骤实现:
1. 获取分片上传预签名地址,可以使用Minio Java SDK提供的`presignedPutObject()`方法实现。具体代码如下:
```java
String objectName = "example-object";
int partNumber = 1;
int partSize = 5 * 1024 * 1024; // 5MB
String uploadId = "example-upload-id";
String presignedUrl = minioClient.presignedPutObject("example-bucket", objectName, 7 * 24 * 60 * 60, null, null, null, partNumber, partSize, uploadId);
```
2. 在后台接口中,可以使用Java的`HttpURLConnection`或者Apache的`HttpClient`等工具类,将分片文件上传到预签名地址。具体代码如下:
```java
URL url = new URL(presignedUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("PUT");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/octet-stream");
FileInputStream inputStream = new FileInputStream(file);
byte[] buffer = new byte[partSize];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
connection.getOutputStream().write(buffer, 0, bytesRead);
}
inputStream.close();
connection.getOutputStream().close();
int responseCode = connection.getResponseCode();
```
3. 在前端中,可以使用`vue-simple-uploader`库进行改造,使用`octet`方式上传分片文件。具体代码如下:
```vue
<template>
<div>
<input type="file" @change="uploadFile">
</div>
</template>
<script>
import SimpleUploader from 'vue-simple-uploader'
export default {
components: {
SimpleUploader
},
methods: {
async uploadFile(event) {
const file = event.target.files[0]
const partSize = 5 * 1024 * 1024 // 5MB
const totalSize = file.size
const totalParts = Math.ceil(totalSize / partSize)
const uploadId = await this.$http.post('/api/minio/initiate-multipart-upload', { filename: file.name })
const parts = []
for (let i = 0; i < totalParts; i++) {
const start = i * partSize
const end = Math.min(start + partSize, totalSize)
const blob = file.slice(start, end)
const presignedUrl = await this.$http.post('/api/minio/presigned-url', { uploadId, partNumber: i + 1 })
const response = await this.$http.put(presignedUrl, blob, { headers: { 'Content-Type': 'application/octet-stream' } })
parts.push({ partNumber: i + 1, etag: response.headers.etag })
}
await this.$http.post('/api/minio/complete-multipart-upload', { uploadId, parts })
}
}
}
</script>
```
阅读全文
相关推荐
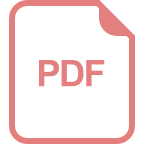
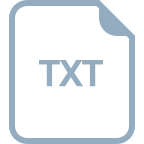
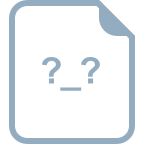


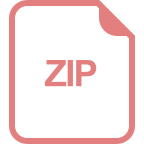
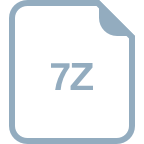
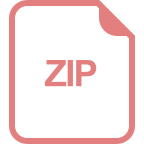
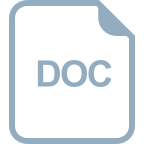









