用java设计一个线段类,其中包括平面坐标相关数据成员,和相关方法,并在主程序中判断三个线段是否组成三角形,如果不行输出各个线段的信息,如果可以输出三角形的面积和周长。
时间: 2024-05-07 11:15:31 浏览: 10
以下是一个示例代码:
```
public class Line {
private double x1, y1, x2, y2;
public Line(double x1, double y1, double x2, double y2) {
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
public double length() {
return Math.sqrt((x2-x1)*(x2-x1) + (y2-y1)*(y2-y1));
}
public boolean isTriangle(Line l2, Line l3) {
double a = this.length();
double b = l2.length();
double c = l3.length();
return (a+b>c) && (a+c>b) && (b+c>a);
}
public double area(Line l2, Line l3) {
double a = this.length();
double b = l2.length();
double c = l3.length();
double s = (a+b+c)/2;
return Math.sqrt(s*(s-a)*(s-b)*(s-c));
}
public double perimeter(Line l2, Line l3) {
return this.length() + l2.length() + l3.length();
}
public void printInfo() {
System.out.println("Line from ("+x1+","+y1+") to ("+x2+","+y2+"), length: "+length());
}
public static void main(String[] args) {
Line l1 = new Line(0,0,2,0);
Line l2 = new Line(2,0,0,2);
Line l3 = new Line(0,2,0,0);
if (l1.isTriangle(l2,l3)) {
double area = l1.area(l2,l3);
double perimeter = l1.perimeter(l2,l3);
System.out.println("Triangle area: "+area+", perimeter: "+perimeter);
} else {
System.out.println("Not a triangle!");
l1.printInfo();
l2.printInfo();
l3.printInfo();
}
}
}
```
这个代码定义了一个 `Line` 类,包括了线段的两个端点的平面坐标和计算线段长度、判断三条线段是否能组成三角形、计算三角形面积和周长等方法。在主程序中,我们定义了三条线段并判断它们是否能组成三角形,如果可以则输出面积和周长,如果不行则输出每条线段的信息。
相关推荐
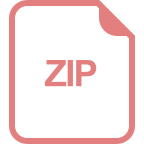


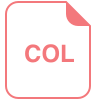
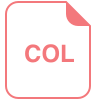
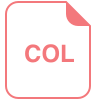









