编程实现单向链表的基本操作: 1.插入元素 2.删除元素 3.获取第i个结点的数据元素 4.判断元素是否存在 5.获取某个元素的下一个元素;C++
时间: 2023-05-26 10:04:14 浏览: 94
typedef struct node {
int data; //数据元素
struct node *next; //指向下一个结点的指针
} Node;
Node *head = NULL; //链表头结点
//在链表末尾插入元素
void insert(int value) {
//创建新结点
Node *new_node = (Node*) malloc(sizeof(Node));
new_node->data = value;
new_node->next = NULL;
//判断链表是否为空
if(head == NULL) {
head = new_node;
return;
}
//遍历链表,找到末尾结点
Node *current = head;
while(current->next != NULL) {
current = current->next;
}
//将新结点插入到末尾
current->next = new_node;
}
//删除值为value的结点
void delete(int value) {
//判断链表是否为空
if(head == NULL) return;
//判断头结点是否为要删除的结点
if(head->data == value) {
Node *temp = head;
head = head->next;
free(temp);
return;
}
//遍历链表,找到要删除的结点
Node *current = head;
while(current->next != NULL && current->next->data != value) {
current = current->next;
}
//判断要删除的结点是否存在
if(current->next == NULL) return;
//将要删除的结点从链表中删除
Node *temp = current->next;
current->next = current->next->next;
free(temp);
}
//获取第i个结点的数据元素
int get(int i) {
//判断链表是否为空
if(head == NULL) return -1;
//遍历链表,找到第i个结点
Node *current = head;
int index = 1;
while(current != NULL && index != i) {
current = current->next;
index++;
}
//判断第i个结点是否存在
if(current == NULL) return -1;
//返回第i个结点的数据元素
return current->data;
}
//判断元素是否存在
int contains(int value) {
//判断链表是否为空
if(head == NULL) return 0;
//遍历链表,查找元素是否存在
Node *current = head;
while(current != NULL) {
if(current->data == value) return 1;
current = current->next;
}
//元素不存在
return 0;
}
//获取值为value的结点的下一个元素
int getNext(int value) {
//判断链表是否为空
if(head == NULL) return -1;
//遍历链表,查找值为value的结点
Node *current = head;
while(current != NULL) {
if(current->data == value) {
//返回下一个结点的数据元素
if(current->next != NULL) return current->next->data;
else return -1;
}
current = current->next;
}
//结点不存在
return -1;
}
阅读全文
相关推荐
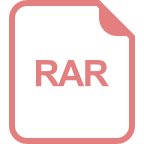




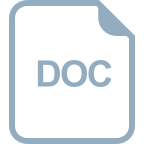
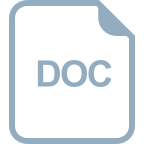
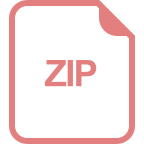
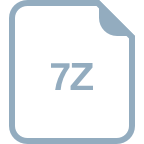
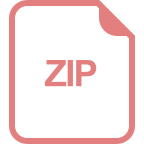
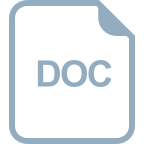
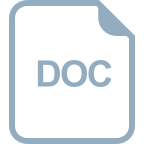
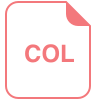
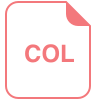
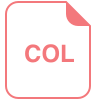
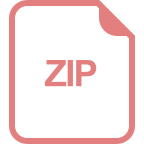
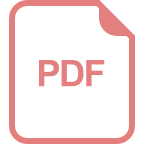