c++ 导出API类 spi类 C#怎么调用
时间: 2023-11-29 08:18:06 浏览: 30
要在 C# 中调用 C++ 导出的 API 类和 SPI 类,需要使用 Platform Invocation Services (P/Invoke)。以下是一些基本步骤:
1. 在 C++ 中将需要导出的类声明为 `extern "C"`,并使用 `__declspec(dllexport)` 指定导出接口。
例如:
```c++
#ifdef __cplusplus
extern "C" {
#endif
__declspec(dllexport) int __stdcall MyFunc(int arg);
#ifdef __cplusplus
}
#endif
```
2. 在 C# 中使用 `[DllImport]` 特性引入 C++ DLL 中的函数或类。
例如:
```c#
using System.Runtime.InteropServices;
[DllImport("MyDLL.dll", CallingConvention = CallingConvention.StdCall)]
public static extern int MyFunc(int arg);
```
3. 如果需要使用 C++ SPI 类,可以在 C# 中定义一个与 C++ 类相同的接口,并使用 `[DllImport]` 引入 C++ DLL 中的类实例化函数和成员函数。
例如:
```c++
class MySPIClass {
public:
virtual void OnEvent(int eventCode) = 0;
};
extern "C" {
__declspec(dllexport) MySPIClass* __stdcall CreateMySPIClassInstance();
__declspec(dllexport) void __stdcall MySPIClass_OnEvent(MySPIClass* instance, int eventCode);
}
```
```c#
[UnmanagedFunctionPointer(CallingConvention.StdCall)]
public delegate void MySPIClass_OnEvent_delegate(int eventCode);
[StructLayout(LayoutKind.Sequential)]
public struct MySPIClass
{
public IntPtr vtbl;
}
[DllImport("MyDLL.dll", CallingConvention = CallingConvention.StdCall)]
public static extern IntPtr CreateMySPIClassInstance();
[DllImport("MyDLL.dll", CallingConvention = CallingConvention.StdCall)]
public static extern void MySPIClass_OnEvent(IntPtr instance, int eventCode);
public static void MySPIClass_OnEvent_wrapper(IntPtr instance, int eventCode)
{
Marshal.GetDelegateForFunctionPointer<MySPIClass_OnEvent_delegate>(Marshal.ReadIntPtr(instance, 0))(eventCode);
}
public static MySPIClass CreateMySPIClass()
{
var instance = new MySPIClass();
instance.vtbl = CreateMySPIClassInstance();
Marshal.WriteIntPtr(instance.vtbl, Marshal.GetFunctionPointerForDelegate(new MySPIClass_OnEvent_delegate(MySPIClass_OnEvent_wrapper)));
return instance;
}
```
这样,就可以在 C# 中调用 C++ 导出的 API 类和 SPI 类了。
相关推荐
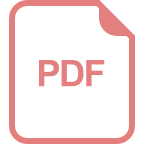














