输入十个学生成绩打印出平均分的同学成绩和学号c++程序
时间: 2023-03-23 08:02:17 浏览: 344
好的,以下是根据您的要求编写的程序,用于输入十个学生的成绩并计算平均分,然后打印出每个学生的成绩和学号c:
```python
# 创建一个字典用于存储学生成绩和学号
students = {}
# 循环10次,输入每个学生的成绩和学号
for i in range(10):
grade = float(input("请输入第{}个学生的成绩:".format(i+1)))
c = input("请输入第{}个学生的学号:".format(i+1))
students[c] = grade
# 计算平均分
average_grade = sum(students.values()) / len(students)
# 打印每个学生的成绩和学号,以及平均分
for c, grade in students.items():
print("学号{}的成绩是{}分".format(c, grade))
print("平均分是{}分".format(average_grade))
```
希望这个程序能够满足您的需求!
相关问题
结构体的应用。要求编写程序,做一个成绩管理系统。 考试结束后,老师登记N个学生的成绩,学生的基本信息包括姓名(chName)、期中成绩(iMiddle)、期末成绩(iFinal)和平均成绩(fAve),请编写程序满足以下要求: 1.使用函数提示用户输入学生成绩,并保存到结构体数组。 2.使用函数计算出每个学生的平均分,将所有学生的信息输出到屏幕上,每个同学的信息占一行。 3.等待用户从键盘输入字符M后,使用函数找出平均分数最高的同学,将其信息打印输出。 4.等待用户从键盘输入一个学生的学号(数组下标号加1),查询输出其信息。 5.等待用户从键盘输入字符P后,使用冒泡排序法根据学生平均成绩从高到底排序后输出。
好的,以下是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义结构体,包括学生基本信息
struct Student {
string chName; // 姓名
int iMiddle; // 期中成绩
int iFinal; // 期末成绩
float fAve; // 平均成绩
};
// 获取学生信息
void getStudentInfo(Student *pStudent, int nCount) {
for (int i = 0; i < nCount; i++) {
cout << "请输入第" << i + 1 << "个学生的姓名、期中成绩、期末成绩(用空格隔开):" << endl;
cin >> pStudent[i].chName >> pStudent[i].iMiddle >> pStudent[i].iFinal;
}
}
// 计算学生平均成绩
void calcAveScore(Student *pStudent, int nCount) {
for (int i = 0; i < nCount; i++) {
pStudent[i].fAve = (pStudent[i].iMiddle + pStudent[i].iFinal) / 2.0;
}
}
// 输出学生信息
void printStudentInfo(Student *pStudent, int nCount) {
cout << "所有学生信息如下:" << endl;
for (int i = 0; i < nCount; i++) {
cout << "姓名:" << pStudent[i].chName << ",期中成绩:" << pStudent[i].iMiddle << ",期末成绩:" << pStudent[i].iFinal << ",平均成绩:" << pStudent[i].fAve << endl;
}
}
// 查找平均分数最高的学生
void findMaxAveScore(Student *pStudent, int nCount) {
float fMaxAveScore = 0;
int nMaxAveScoreIndex = 0;
for (int i = 0; i < nCount; i++) {
if (pStudent[i].fAve > fMaxAveScore) {
fMaxAveScore = pStudent[i].fAve;
nMaxAveScoreIndex = i;
}
}
cout << "平均分数最高的学生信息如下:" << endl;
cout << "姓名:" << pStudent[nMaxAveScoreIndex].chName << ",期中成绩:" << pStudent[nMaxAveScoreIndex].iMiddle << ",期末成绩:" << pStudent[nMaxAveScoreIndex].iFinal << ",平均成绩:" << pStudent[nMaxAveScoreIndex].fAve << endl;
}
// 根据平均成绩排序(冒泡排序)
void sortStudentByAveScore(Student *pStudent, int nCount) {
for (int i = 0; i < nCount - 1; i++) {
for (int j = 0; j < nCount - i - 1; j++) {
if (pStudent[j].fAve < pStudent[j + 1].fAve) {
swap(pStudent[j], pStudent[j + 1]);
}
}
}
}
// 根据学号查询学生信息
void findStudentByIndex(Student *pStudent, int nCount) {
int nIndex = 0;
cout << "请输入要查询的学生的学号(数组下标号加1):" << endl;
cin >> nIndex;
if (nIndex <= 0 || nIndex > nCount) {
cout << "输入的学号不合法,请重新输入!" << endl;
findStudentByIndex(pStudent, nCount);
return;
}
cout << "学号为" << nIndex << "的学生信息如下:" << endl;
cout << "姓名:" << pStudent[nIndex - 1].chName << ",期中成绩:" << pStudent[nIndex - 1].iMiddle << ",期末成绩:" << pStudent[nIndex - 1].iFinal << ",平均成绩:" << pStudent[nIndex - 1].fAve << endl;
}
int main() {
int nCount = 0;
cout << "请输入学生的个数:" << endl;
cin >> nCount;
// 动态分配内存
Student *pStudent = new Student[nCount];
// 获取学生信息
getStudentInfo(pStudent, nCount);
// 计算学生平均成绩
calcAveScore(pStudent, nCount);
// 输出学生信息
printStudentInfo(pStudent, nCount);
// 查找平均分数最高的学生
char cFindMax = ' ';
cout << "请输入字符M,查找平均分数最高的学生:" << endl;
cin >> cFindMax;
if (cFindMax == 'M') {
findMaxAveScore(pStudent, nCount);
}
// 根据平均成绩排序
char cSort = ' ';
cout << "请输入字符P,按平均成绩从高到低排序并输出:" << endl;
cin >> cSort;
if (cSort == 'P') {
sortStudentByAveScore(pStudent, nCount);
printStudentInfo(pStudent, nCount);
}
// 根据学号查询学生信息
char cFindIndex = ' ';
cout << "请输入字符S,查询学生信息:" << endl;
cin >> cFindIndex;
if (cFindIndex == 'S') {
findStudentByIndex(pStudent, nCount);
}
// 释放内存
delete[] pStudent;
return 0;
}
```
注意:在程序中使用了动态分配内存的方式来创建结构体数组,使用完后需要释放内存,否则会出现内存泄漏的问题。
用c++写一个班上有30名学生,每个学生的数据作为一个记录,每个记录包括学号、姓名、三门课程的成绩和三门课程平均成绩。从键盘输入学生的学号、姓名及三门课。的代码
在C++中,你可以创建一个结构体来表示学生的信息,并使用数组来存储所有学生的数据。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
// 定义一个结构体表示学生信息
struct Student {
int id;
std::string name;
float course1_score, course2_score, course3_score; // 三门课程的成绩
float average_score; // 平均成绩,初始化为0,待计算
};
int main() {
const int total_students = 30;
Student students[total_students]; // 存储所有学生信息的数组
// 循环读取每位学生的数据
for (int i = 0; i < total_students; ++i) {
std::cout << "请输入第" << (i + 1) << "位同学的学号: ";
std::cin >> students[i].id;
std::cout << "请输入第" << (i + 1) << "位同学的姓名: ";
std::cin >> students[i].name;
std::cout << "请输入第" << (i + 1) << "位同学第一门课程的成绩: ";
std::cin >> students[i].course1_score;
std::cout << "请输入第" << (i + 1) << "位同学第二门课程的成绩: ";
std::cin >> students[i].course2_score;
std::cout << "请输入第" << (i + 1) << "位同学第三门课程的成绩: ";
std::cin >> students[i].course3_score;
// 计算平均成绩并存入结构体
students[i].average_score = (students[i].course1_score + students[i].course2_score + students[i].course3_score) / 3.0;
}
// 打印部分学生信息作为例子
for (int i = 0; i < 5; ++i) { // 输出前5位学生的数据
std::cout << "学号: " << students[i].id << ", 姓名: " << students[i].name << ", 平均成绩: " << students[i].average_score << "\n";
}
return 0;
}
```
在这个程序中,我们首先定义了一个`Student`结构体,然后使用循环逐个输入每个学生的信息。最后,我们展示了如何简单地打印出部分学生的数据。
阅读全文
相关推荐
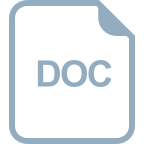
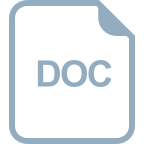
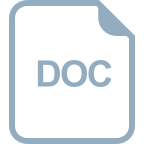
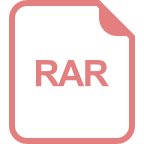
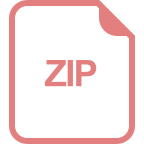

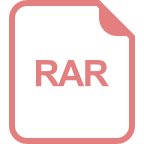
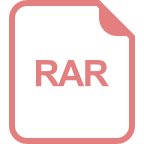
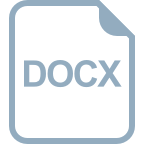
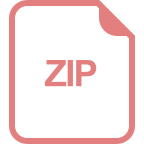
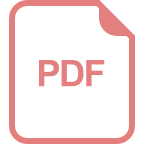
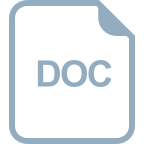
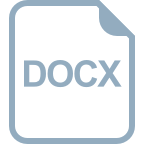
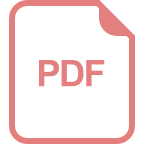
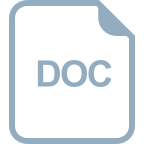
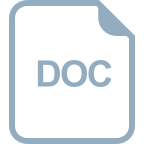
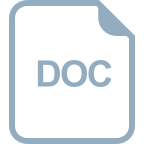