写一段傅里叶变换算法的java代码
时间: 2023-08-31 20:22:05 浏览: 57
### 回答1:
傅里叶变换是用来对一个信号进行频率分析的算法。下面是一段使用Java实现的傅里叶变换的代码示例:
```
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
public class FFT {
private final int N;
private final List<Complex> roots;
private final Function<Complex, Complex> inverse;
public FFT(int n, boolean inverse) {
this.N = n;
this.roots = roots(n, inverse);
this.inverse = inverse ? Complex::conjugate : Function.identity();
}
public List<Complex> apply(List<Complex> a) {
int n = a.size();
if (n != N) {
throw new IllegalArgumentException("Size mismatch");
}
if (n == 1) {
return a;
}
List<Complex> even = new ArrayList<>(n / 2);
List<Complex> odd = new ArrayList<>(n / 2);
for (int i = 0; i < n; i++) {
if (i % 2 == 0) {
even.add(a.get(i));
} else {
odd.add(a.get(i));
}
}
List<Complex> q = apply(even);
List<Complex> r = apply(odd);
List<Complex> y = new ArrayList<>(n);
for (int k = 0; k < n / 2; k++) {
Complex z = roots.get(k).multiply(r.get(k));
y.add(q.get(k).add(z));
y.add(q.get(k).subtract(z));
}
return y;
}
public static List<Complex> roots(int n, boolean inverse) {
List<Complex> roots = new ArrayList<>(n / 2);
double angle = 2 * Math.PI / n * (inverse ? -1 : 1);
for (int k = 0; k < n / 2; k++) {
roots.add(Complex.fromPolar(1, angle * k));
}
return roots;
}
}
class Complex {
final double re;
final double im;
public Complex(double re, double im) {
this.re = re;
this.im = im;
}
public static Complex fromPolar(double r, double theta) {
return new Complex(r * Math.cos(theta), r * Math.sin(theta));
}
public double re() {
return re;
}
public double im() {
return im;
}
public Complex conjugate() {
return new Complex(re, -im);
}
public Complex add
### 回答2:
傅里叶变换是信号处理领域中常用的算法,可将时域信号转换为频域信号。以下是一个简单的傅里叶变换算法的Java代码示例:
```java
import java.util.Arrays;
public class FourierTransform {
// 傅里叶变换算法
public static Complex[] fft(Complex[] x) {
int N = x.length;
// 基本情况,如果输入只有一个元素,则直接返回
if (N == 1) {
return new Complex[] { x[0] };
}
// 创建偶数索引和奇数索引的数组
Complex[] even = new Complex[N / 2];
Complex[] odd = new Complex[N / 2];
for (int k = 0; k < N / 2; k++) {
even[k] = x[2 * k];
odd[k] = x[2 * k + 1];
}
// 递归计算偶数索引和奇数索引的傅里叶变换
Complex[] q = fft(even);
Complex[] r = fft(odd);
// 合并两个结果
Complex[] y = new Complex[N];
for (int k = 0; k < N / 2; k++) {
double kth = -2 * k * Math.PI / N;
Complex wk = new Complex(Math.cos(kth), Math.sin(kth));
y[k] = q[k].plus(wk.times(r[k]));
y[k + N / 2] = q[k].minus(wk.times(r[k]));
}
return y;
}
public static void main(String[] args) {
Complex[] x = new Complex[] { new Complex(1, 0), new Complex(2, 0), new Complex(3, 0), new Complex(4, 0) };
// 调用傅里叶变换算法
Complex[] y = fft(x);
System.out.println("输入信号:");
System.out.println(Arrays.toString(x));
System.out.println("傅里叶变换结果:");
System.out.println(Arrays.toString(y));
}
}
class Complex {
private final double real;
private final double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex plus(Complex other) {
double real = this.real + other.real;
double imag = this.imag + other.imag;
return new Complex(real, imag);
}
public Complex minus(Complex other) {
double real = this.real - other.real;
double imag = this.imag - other.imag;
return new Complex(real, imag);
}
public Complex times(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
@Override
public String toString() {
return "(" + real + ", " + imag + ")";
}
}
```
以上代码实现了一个简单的傅里叶变换算法,在`fft()`方法中,使用递归将输入数组分成偶数和奇数索引两个子数组,分别对其进行傅里叶变换,然后合并两个结果,最终得到傅里叶变换结果。代码中使用的`Complex`类用于表示复数,并实现了复数的加、减、乘等运算。在`main()`方法中,首先创建一个复数数组作为输入信号,然后调用傅里叶变换算法计算结果,最后输出结果。
相关推荐
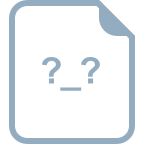














