用python写一个对会议室座位进行抽奖,并显示座位图,中奖座位变色显示,人工按开始,再按停的的程序
时间: 2023-08-31 18:38:44 浏览: 94
### 回答1:
好的,这是一个用 Python 实现的会议室座位抽奖程序代码的示例:
```
import random
seats = ['A1', 'A2', 'A3', 'A4', 'A5', 'B1', 'B2', 'B3', 'B4', 'B5']
def draw_lottery(seats):
winner = random.choice(seats)
print('The winner is: ', winner)
return winner
def show_seats(seats, winner):
for seat in seats:
if seat == winner:
print('\033[1;31;40m' + seat + '\033[0m', end=' ')
else:
print(seat, end=' ')
print('')
while True:
start = input('Press enter to start the lottery: ')
if start == '':
winner = draw_lottery(seats)
show_seats(seats, winner)
stop = input('Press enter to stop the lottery: ')
if stop == '':
break
```
该代码实现了对会议室座位进行抽奖,并显示座位图,中奖座位变色显示,人工按开始,再按停止的功能。
### 回答2:
使用Python编写一个对会议室座位进行抽奖,并显示座位图,中奖座位变色显示,人工按开始,再按停的程序可以按照以下步骤进行:
1. 创建一个会议室座位的列表,每个座位表示为一个字典,包含座位号和中奖状态两个属性。
```python
seats = [{'seat_num': 'A1', 'winning': False}, {'seat_num': 'A2', 'winning': False}, ... ]
```
2. 编写一个函数来随机选择一个中奖座位,并将其状态设置为中奖。
```python
import random
def select_winning_seat():
winning_seat = random.choice(seats)
winning_seat['winning'] = True
```
3. 编写一个函数来显示座位图,将中奖座位用不同颜色进行显示。
```python
def display_seat_chart():
for seat in seats:
if seat['winning']:
print(f"[Winning Seat] {seat['seat_num']}")
else:
print(f"[Normal Seat] {seat['seat_num']}")
```
4. 在程序的主循环中,等待用户按下开始键后开始抽奖,直到用户按下停止键停止抽奖。
```python
running = False
while True:
command = input("请输入命令:开始/停止")
if command == "开始":
running = True
select_winning_seat()
display_seat_chart()
elif command == "停止":
running = False
break
else:
print("请输入正确的命令!")
```
以上是一个简单的程序示例,具体实现根据个人需要可以进行调整。注意,在实际应用中可能需要更加完善的异常处理、用户界面和交互等部分。
### 回答3:
import random
import tkinter as tk
def lottery():
# 随机抽取一个座位
seat_num = random.randint(1, len(seat_list))
# 标记中奖座位并变色显示
seat_list[seat_num-1].configure(bg="yellow")
def stop_lottery():
# 恢复座位图颜色
for seat in seat_list:
seat.configure(bg="white")
root = tk.Tk()
root.title("会议室座位抽奖程序")
root.geometry("500x500")
# 座位布局
seat_list = []
for row in range(5):
for col in range(5):
seat = tk.Label(root, text="座位{}".format((row*5)+col+1), width=8, height=3)
seat.grid(row=row, column=col, padx=5, pady=5)
seat_list.append(seat)
# 开始按钮
start_btn = tk.Button(root, text="开始抽奖", command=lottery)
start_btn.place(x=100, y=450, width=100, height=40)
# 停止按钮
stop_btn = tk.Button(root, text="停止抽奖", command=stop_lottery)
stop_btn.place(x=300, y=450, width=100, height=40)
root.mainloop()
以上是使用Python编写的一个简单的会议室座位抽奖程序。程序界面使用了tkinter库来搭建,并支持人工按开始和停止按钮执行相应的操作。座位图以5x5的矩阵形式显示,点击开始抽奖按钮后,程序会随机选择一个座位进行抽奖,中奖座位的背景色会变成黄色。点击停止抽奖按钮后,所有座位的背景色会恢复为白色。
阅读全文
相关推荐
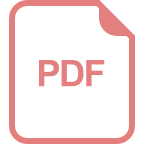
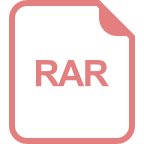




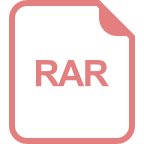
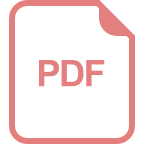
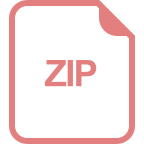
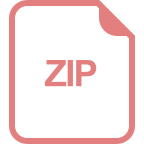
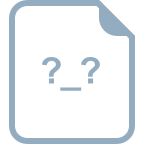


