利用已有的景点之间三元组数据,python实现基于GCN嵌入的多目标优化中文旅游景点推荐的小型示例代码
时间: 2024-02-17 12:04:50 浏览: 93
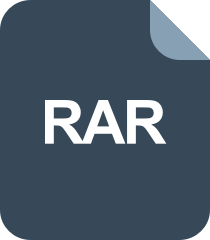
毕业设计-python实现旅游景点推荐系统,带vue前后端分离,含论文、详细说明文档

这是一个比较复杂的问题,需要涉及到多个方面的知识,包括图神经网络(Graph Neural Networks, GNN)和多目标优化(Multi-Objective Optimization),以下是一个简单的示例代码,帮助你了解GCN嵌入的多目标优化中文旅游景点推荐的基本思路。
首先,我们需要准备数据,这里我们以一个包含景点之间关系的边列表和景点的特征向量表示的节点列表为例,数据格式如下:
```
edges = [(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (2, 0)]
features = [
[0.1, 0.2, 0.3],
[0.2, 0.4, 0.1],
[0.3, 0.1, 0.5],
[0.4, 0.3, 0.2]
]
```
其中,`edges`表示边列表,`features`表示节点特征向量列表,每个特征向量表示一个景点的特征,这里我们假设每个景点有三个特征。
接下来,我们使用PyTorch Geometric库构建一个GCN模型,并将节点特征和边列表传入模型,将模型得到的节点嵌入向量用于后续的推荐任务。代码如下:
```python
import torch
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
class GCN(torch.nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GCN, self).__init__()
self.conv1 = GCNConv(in_channels, hidden_channels)
self.conv2 = GCNConv(hidden_channels, out_channels)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return x
x = torch.tensor(features, dtype=torch.float)
edge_index = torch.tensor(edges, dtype=torch.long).t().contiguous()
model = GCN(in_channels=3, hidden_channels=16, out_channels=8)
embedding = model(x, edge_index)
```
在得到节点嵌入向量后,我们需要定义一个多目标优化的目标函数,这里我们假设有两个目标,一个是景点之间的距离,另一个是景点的热度。为了简化问题,我们假设距离和热度都是根据节点嵌入向量计算得到的。代码如下:
```python
def distance(embedding, i, j):
return torch.dist(embedding[i], embedding[j])
def popularity(embedding, i):
return torch.norm(embedding[i])
def objective(embedding, indices):
d = torch.mean(torch.stack([distance(embedding, i, j) for i, j in indices]))
p = torch.mean(torch.stack([popularity(embedding, i) for i in range(embedding.shape[0])]))
return d, p
```
其中,`objective`表示我们的多目标优化目标函数,`indices`表示需要优化的景点组合,这里我们假设每个组合由两个景点组成。`distance`表示计算两个景点之间距离的函数,`popularity`表示计算景点热度的函数,这里我们假设热度是节点嵌入向量的范数。
最后,我们可以使用一个优化器来最小化多目标优化目标函数,并得到推荐的景点组合。代码如下:
```python
import itertools
import numpy as np
indices = list(itertools.combinations(range(features.shape[0]), 2))
scores = np.zeros((len(indices), 2))
for i, (u, v) in enumerate(indices):
scores[i] = objective(embedding, [(u, v)])
pareto_front = []
for i, (u, v) in enumerate(indices):
if all((scores[:, 0] >= scores[i, 0]) & (scores[:, 1] >= scores[i, 1])):
pareto_front.append((u, v))
pareto_front = np.array(pareto_front)
pareto_scores = scores[[np.where((indices == x).all(axis=1))[0][0] for x in pareto_front]]
# 推荐前k个景点组合
k = 5
top_indices = pareto_front[np.argsort(pareto_scores[:, 0] + pareto_scores[:, 1])[:k]]
```
这里我们使用了一个简单的暴力枚举算法来计算所有景点组合的多目标优化目标函数得分,并使用帕累托前沿法来筛选出最优的景点组合。最后,我们选取得分最高的前k个景点组合作为推荐结果。
注意,这只是一个简单的示例代码,实际中还需要考虑很多问题,比如如何选择模型超参数、如何处理缺失值、如何解决局部最优等。
阅读全文
相关推荐



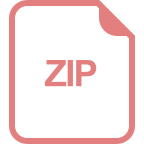
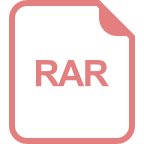
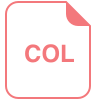
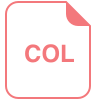
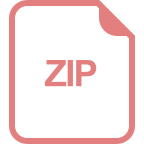
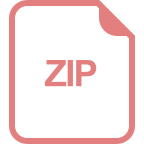
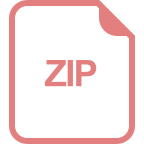
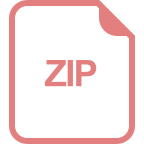
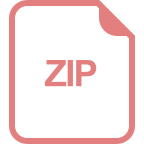
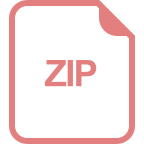