某班有10个学生的,编写一个函数inputscore,实现从键盘上录入10名学生的成绩,存入数组中
时间: 2024-05-12 11:14:19 浏览: 81
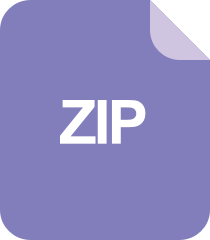
接收从键盘输入某班学生的学号、姓名、成绩,对学 生的成绩进行排序.zip

下面是一个简单的 Python 函数 `inputscore`,可以实现从键盘上录入10名学生的成绩,并将它们存储到一个数组中:
```python
def inputscore():
scores = [] # 创建一个空列表,用于存储成绩
for i in range(10):
score = input("请输入第%d个学生的成绩:" % (i+1))
scores.append(float(score)) # 将成绩转换为浮点数并添加到列表中
return scores
```
使用方法:
```python
scores = inputscore()
print(scores)
```
运行程序后,依次输入每个学生的成绩,最后会将这些成绩存储到一个长度为10的数组 `scores` 中,并输出该数组的内容。
阅读全文
相关推荐








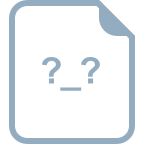





