#include<iostream> #include<string> using namespace std; class shape { public: double width; double length; shape(double width=0,double length=0) :width(width), length(length){} }; class Rectangle :public shape { public: double get_Aera() { return (width * length); } Rectangle(double width = 0, double length = 0) {} }; struct Student { string name; int age; double score; Student(string name="",int age=0,double score=0):name(name),age(age),score(score){} }; int main() { Rectangle r(3.4,4.5); cout << r.get_Aera() << endl; Student s("Tom", 20, 90); cout << s.name << s.age << s.score << endl; return 0; }改进这段代码
时间: 2023-11-22 12:53:43 浏览: 39
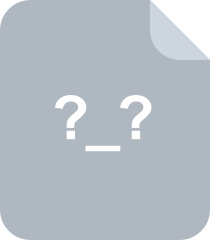
#include <iostream>
这段代码可以改进的地方如下:
1. 在 Rectangle 类的构造函数中没有对基类的构造函数进行调用,可以在子类的构造函数中调用父类的构造函数来初始化基类的成员变量。
2. 在打印学生信息时,缺少分隔符,可以在输出时加上分隔符。
改进后的代码如下:
```cpp
#include<iostream>
#include<string>
using namespace std;
class shape {
public:
double width;
double length;
shape(double width = 0, double length = 0)
:width(width), length(length){}
};
class Rectangle :public shape {
public:
double get_Aera() {
return (width * length);
}
Rectangle(double width = 0, double length = 0)
:shape(width, length){}
};
struct Student {
string name;
int age;
double score;
Student(string name="",int age=0,double score=0)
:name(name),age(age),score(score){}
};
int main() {
Rectangle r(3.4,4.5);
cout << "矩形面积为:" << r.get_Aera() << endl;
Student s("Tom", 20, 90);
cout << "学生信息:" << s.name << ", " << s.age << ", " << s.score << endl;
return 0;
}
```
阅读全文
相关推荐
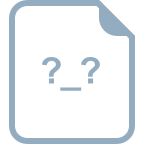



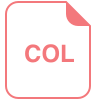






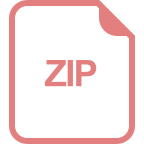
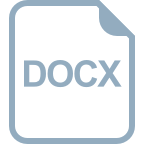
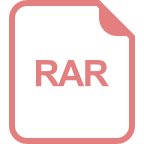
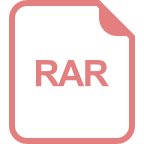
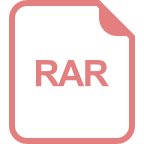