python设计一个类,实现向量的加、减、内积等操作,需要判断输入的向量是否都是数值,并且判断向量长度是否一致
时间: 2024-05-11 09:19:22 浏览: 101
可以使用以下代码实现:
```python
class Vector:
def __init__(self, *args):
self.values = args
self.length = len(args)
self.check_input()
def check_input(self):
for value in self.values:
if not isinstance(value, (int, float)):
raise TypeError('Vector values must be numeric')
if self.length == 0:
raise ValueError('Vector must have at least one value')
def __add__(self, other):
if self.length != other.length:
raise ValueError('Vectors must have the same length')
return Vector(*[self.values[i] + other.values[i] for i in range(self.length)])
def __sub__(self, other):
if self.length != other.length:
raise ValueError('Vectors must have the same length')
return Vector(*[self.values[i] - other.values[i] for i in range(self.length)])
def dot(self, other):
if self.length != other.length:
raise ValueError('Vectors must have the same length')
return sum([self.values[i] * other.values[i] for i in range(self.length)])
```
这个类接受任意数量的数值作为参数,并且会检查输入是否都是数值。向量的加法和减法会检查两个向量长度是否一致,如果不一致则会抛出异常。内积操作也会检查向量长度是否一致。
阅读全文
相关推荐
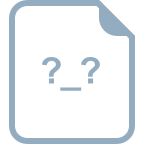

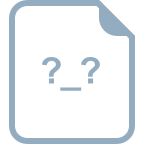
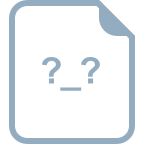
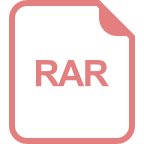
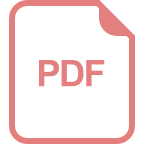
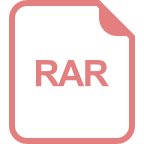
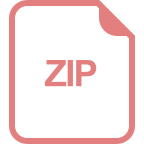
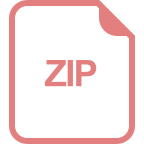
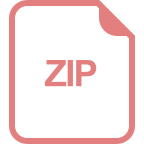
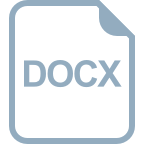
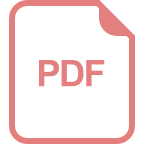
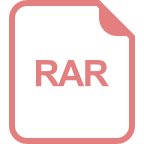
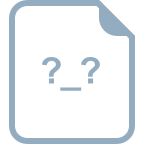
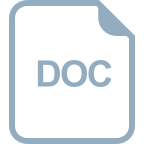
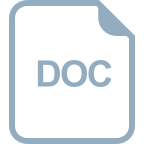
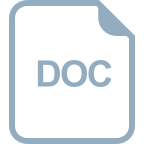