设计一个类,实现向量的加、减、内积等操作,需要判断输入的向量是否都是数值,并且判断向量长度是否一致。 例如(1,2,3)+(3,4,5
时间: 2023-10-20 14:07:13 浏览: 40
)=(4,6,8),向量内积为1*3+2*4+3*5=22。
以下是一个基本的向量类的实现示例:
```python
class Vector:
def __init__(self, vec):
self.vec = vec
def __str__(self):
return f"{self.vec}"
def __add__(self, other):
if isinstance(other, Vector) and len(self.vec) == len(other.vec):
return Vector([self.vec[i] + other.vec[i] for i in range(len(self.vec))])
else:
raise ValueError("Invalid input, input should be a vector with same length.")
def __sub__(self, other):
if isinstance(other, Vector) and len(self.vec) == len(other.vec):
return Vector([self.vec[i] - other.vec[i] for i in range(len(self.vec))])
else:
raise ValueError("Invalid input, input should be a vector with same length.")
def __mul__(self, other):
if isinstance(other, Vector) and len(self.vec) == len(other.vec):
return sum([self.vec[i] * other.vec[i] for i in range(len(self.vec))])
else:
raise ValueError("Invalid input, input should be a vector with same length.")
```
其中,`__init__` 方法用于初始化向量类,`__str__` 方法将向量转换为字符串输出,`__add__` 方法实现向量加法,`__sub__` 方法实现向量减法,`__mul__` 方法实现向量内积。
使用示例:
```python
v1 = Vector([1, 2, 3])
v2 = Vector([3, 4, 5])
print(v1 + v2) # output: [4, 6, 8]
print(v1 - v2) # output: [-2, -2, -2]
print(v1 * v2) # output: 22
```
相关推荐
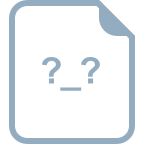
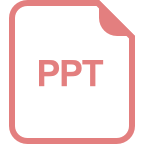














