用vue2实现飞书日历的拖拽选择区域创建日程的功能
时间: 2023-12-30 17:08:32 浏览: 39
要实现飞书日历的拖拽选择区域创建日程的功能,可以参考以下步骤:
1. 在 Vue 组件中引入飞书日历组件,并将其包裹在一个容器中。
2. 给容器绑定 mousedown 事件,记录鼠标按下时的坐标。
3. 给容器绑定 mousemove 事件,计算出当前鼠标位置与按下时的坐标之间的距离,并将其作为拖拽选择区域的宽和高。
4. 在容器中动态生成一个矩形框,用于表示拖拽选择区域。
5. 给容器绑定 mouseup 事件,在该事件中根据矩形框的位置和大小计算出拖拽选择的时间范围,并将其传递给飞书日历组件中的创建日程方法。
下面是一个示例代码,实现了飞书日历的拖拽选择区域创建日程的功能:
```html
<template>
<div class="calendar-container" @mousedown="handleMouseDown" @mousemove="handleMouseMove" @mouseup="handleMouseUp">
<feishu-calendar :events="events" />
<div v-show="isDragging" class="drag-box" :style="{ width: dragWidth + 'px', height: dragHeight + 'px', top: dragTop + 'px', left: dragLeft + 'px' }"></div>
</div>
</template>
<script>
import FeishuCalendar from 'feishu-calendar';
export default {
components: {
FeishuCalendar,
},
data() {
return {
events: [
// 日程列表
],
isDragging: false,
startX: 0,
startY: 0,
endX: 0,
endY: 0,
dragLeft: 0,
dragTop: 0,
dragWidth: 0,
dragHeight: 0,
};
},
methods: {
handleMouseDown(e) {
this.startX = e.clientX;
this.startY = e.clientY;
this.isDragging = true;
},
handleMouseMove(e) {
if (this.isDragging) {
this.endX = e.clientX;
this.endY = e.clientY;
this.dragWidth = Math.abs(this.endX - this.startX);
this.dragHeight = Math.abs(this.endY - this.startY);
this.dragLeft = Math.min(this.startX, this.endX);
this.dragTop = Math.min(this.startY, this.endY);
}
},
handleMouseUp() {
if (this.isDragging) {
this.isDragging = false;
const start = this.$refs.feishuCalendar.getDateTimeByPosition({ x: this.dragLeft, y: this.dragTop });
const end = this.$refs.feishuCalendar.getDateTimeByPosition({ x: this.dragLeft + this.dragWidth, y: this.dragTop + this.dragHeight });
const event = {
start: start.toISOString(),
end: end.toISOString(),
title: '',
};
this.events.push(event);
}
},
},
};
</script>
<style>
.calendar-container {
position: relative;
}
.drag-box {
position: absolute;
border: 2px dashed #aaa;
opacity: 0.5;
pointer-events: none;
}
</style>
```
在上面的代码中,我们通过引入飞书日历组件 `FeishuCalendar`,并在容器中动态生成一个矩形框 `div.drag-box`,实现了拖拽选择区域创建日程的功能。在 `handleMouseDown` 方法中,记录鼠标按下时的坐标,并将 `isDragging` 置为 `true`,表示正在拖拽。在 `handleMouseMove` 方法中,根据鼠标移动的距离计算出拖拽选择区域的位置和大小,并动态更新矩形框的样式。在 `handleMouseUp` 方法中,根据矩形框的位置和大小计算出拖拽选择的时间范围,并将其作为一个新的日程添加到日程列表中。
相关推荐
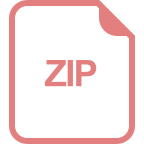










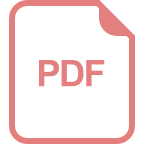